Google Autocomplete API
The Google Autocomplete API can be accessed using the /api/v1/search?engine=google_autocomplete
API endpoint to scrape real-time results.
API Parameters
Search Query
-
- Name
-
q
- Required
- Required
- Description
-
Search query that would produce autocomplete suggestions.
Filters
-
- Name
-
client
- Required
- Optional
- Description
-
This parameter identifies the client initiating the autocomplete request. For instance, using
chrome
as the value signifies that the request comes from the Chrome browser. The API may customize the response in terms of format or content based on the identified client. Various other values can be set to represent different browsers or applications, each affecting the autocomplete results in a corresponding manner. Key client types supported by this API include:chrome
(default) - used for Chrome browser searches.chrome-omni
- used for Chrome address bar.gws-wiz
- used for Chrome Google homepage.gws-wiz-local
- used for searches on Google Local.safari
- used when Google is opened in Safari browserfirefox
- used for Firefox browser searches.psy-ab
- used for Google search queries.youtube
- origin unknown. Returns JSONP.toolbar
- origin unknown. Returns XML.
-
- Name
-
cp
- Required
- Optional
- Description
-
The
cp
parameter is used to determine the cursor position within the search query for autocomplete requests. A0
value places the cursor at the start of the query (like|some query
), whereas not includingcp
suggests the cursor is at the end of the query (likesome query|
). The location of the cursor is important as it affects the suggestions provided by the autocomplete feature of the API.
Localization
-
- Name
-
gl
- Required
- Optional
- Description
-
The default parameter
us
defines the country of the search. Check the full list of supported Googlegl
countries.
-
- Name
-
hl
- Required
- Optional
- Description
-
The default parameter
en
defines the interface language of the search. Check the full list of supported Googlehl
languages.
Engine
-
- Name
-
engine
- Required
- Required
- Description
-
Parameter defines an engine that will be used to retrieve real-time data. It must be set to
google_autocomplete
.
API key
-
- Name
-
api_key
- Required
- Required
- Description
-
The
api_key
authenticates your requests. Use it as a query parameter (https://www.searchapi.io/api/v1/search?api_key=YOUR_API_KEY
) or in the Authorization header (Bearer YOUR_API_KEY
).
API Examples
Google Home Page Results
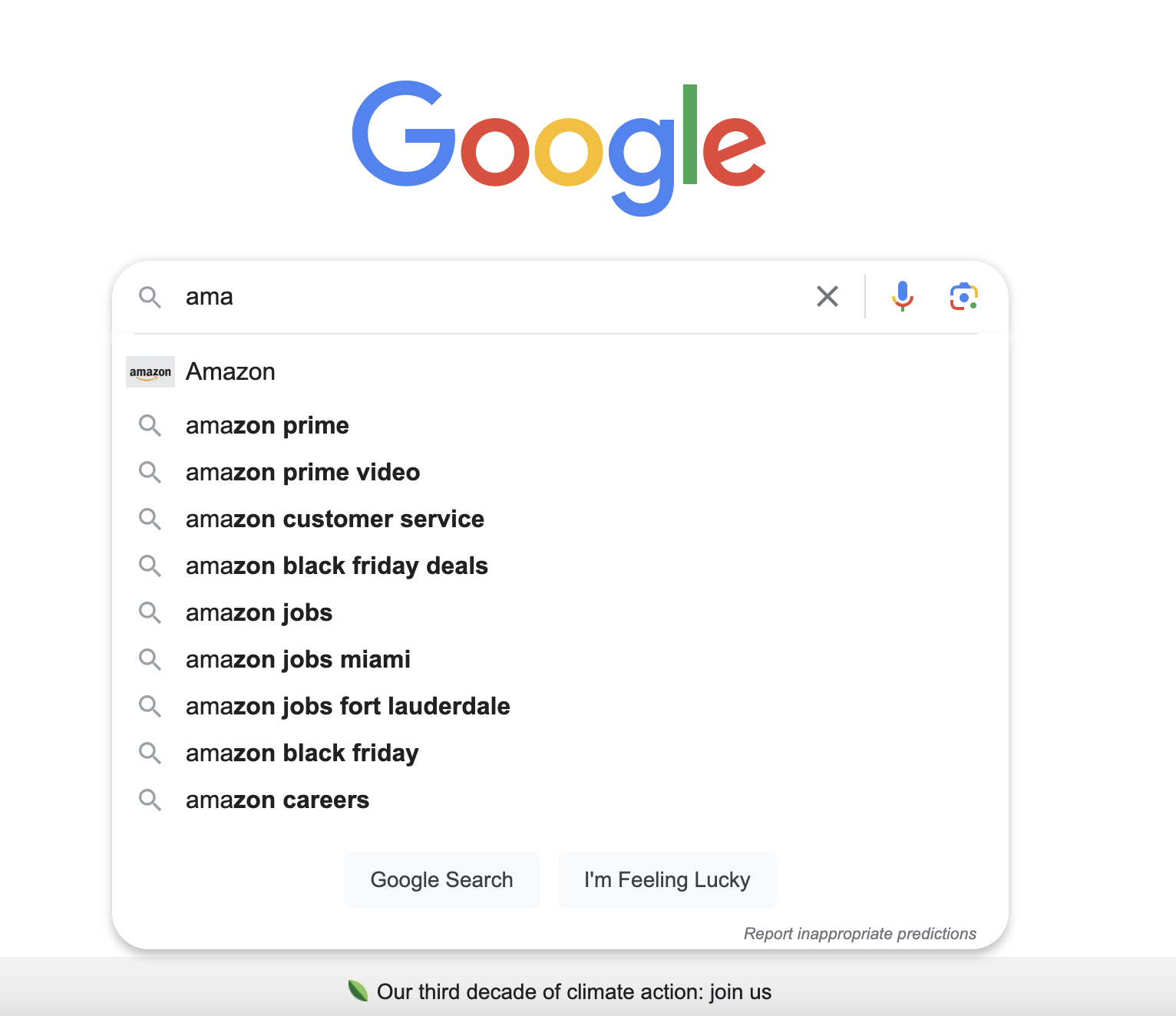
Modifying client
parameters impacts the results of completions. An example is provided for gws-wiz
.
https://www.searchapi.io/api/v1/search?client=gws-wiz&engine=google_autocomplete&q=ama
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_autocomplete",
"q": "ama",
"client": "gws-wiz"
}
response = requests.get(url, params=params)
print(response.text)
{
"suggestions": [
{
"value": "amazon",
"type": 46,
"title": "Amazon",
"thumbnail": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTPmIckKCzf8qUxF89dXVgIkQQOaAAVW9PwzBh_I9E&s=10"
},
{ "value": "amazon prime" },
{ "value": "amazon prime video" },
...
]
}
Safari Results
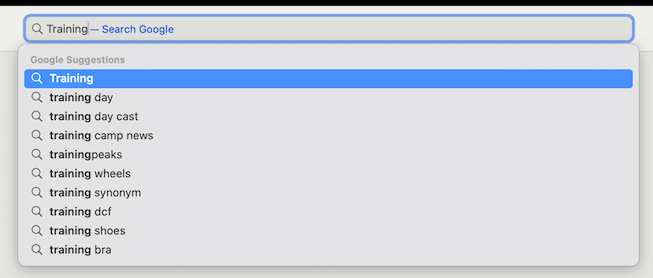
Modifying client
parameters impacts the results of completions. An example is provided for safari
.
https://www.searchapi.io/api/v1/search?client=safari&engine=google_autocomplete&q=Training
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_autocomplete",
"q": "Training",
"client": "safari"
}
response = requests.get(url, params=params)
print(response.text)
{
"suggestions": [
{ "value": "training" },
{ "value": "training day" },
...
]
}
Chrome Address Bar Results
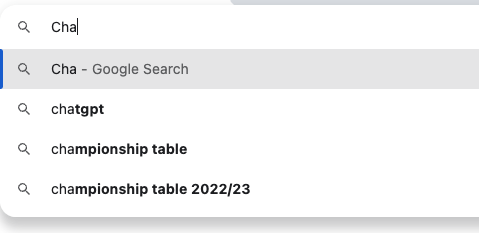
Modifying client
parameters impacts the results of completions. An example is provided for chrome-omni
.
https://www.searchapi.io/api/v1/search?client=chrome-omni&engine=google_autocomplete&q=Cha
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_autocomplete",
"q": "Cha",
"client": "chrome-omni"
}
response = requests.get(url, params=params)
print(response.text)
{
"suggestions": [
{
"value": "chatgpt",
"relevance": 801,
"type": "QUERY"
},
...
],
"verbatim_relevance": 851
}
Custom Cursor Pointer
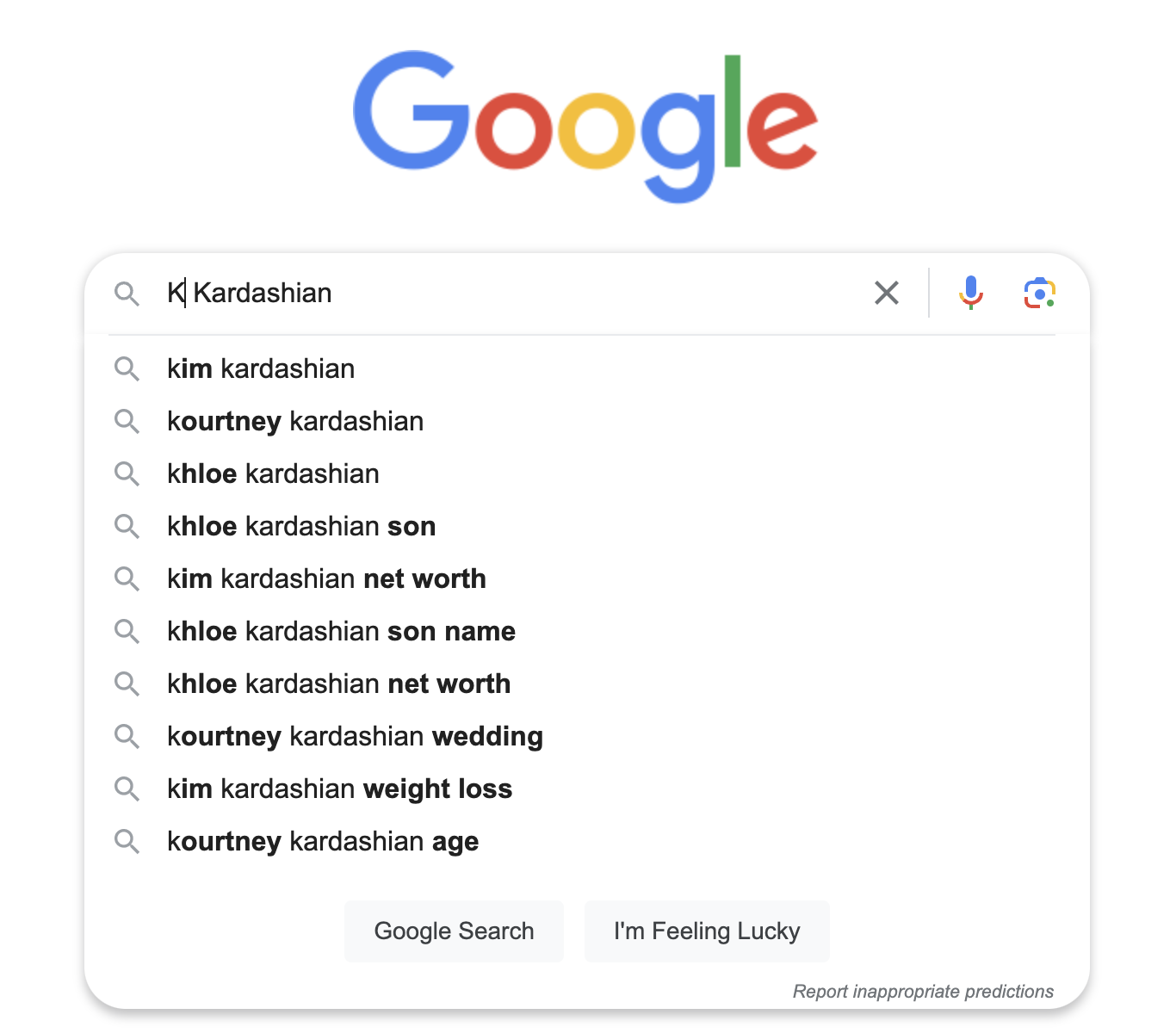
Changing cp
parameter impacts suggestions. Setting cp
parameter to 1
will move the cursor pointer one position to the right.
https://www.searchapi.io/api/v1/search?client=gws-wiz&cp=1&engine=google_autocomplete&q=K+Kardashian
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_autocomplete",
"q": "K Kardashian",
"client": "gws-wiz",
"cp": "1"
}
response = requests.get(url, params=params)
print(response.text)
{
"suggestions": [
{ "value": "kim kardashian" },
{ "value": "kourtney kardashian" },
...
]
}
Language & Location set to Spanish Results
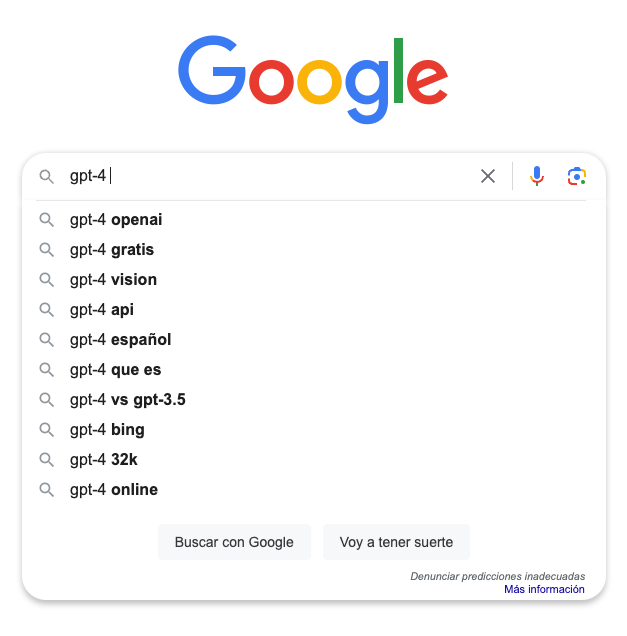
Modifying gl
& hl
parameters impacts the results of completions. An example is provided for es
.
https://www.searchapi.io/api/v1/search?client=gws-wiz&engine=google_autocomplete&gl=es&hl=es&q=gpt-4
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_autocomplete",
"q": "gpt-4",
"client": "gws-wiz",
"gl": "es",
"hl": "es"
}
response = requests.get(url, params=params)
print(response.text)
{
"suggestions": [
{ "value": "gpt-4 openai" },
{ "value": "gpt-4 gratis" },
...
]
}