Google Play Store API
Google Play Store API uses /api/v1/search?engine=google_play_store
API endpoint to scrape real-time results
API Parameters
Categories
-
- Name
-
store
- Required
- Required
- Description
-
Parameter defines the store you would like to retrieve. There are several options to pick:
apps
,games
,movies
, andbooks
.
-
- Name
-
chart
- Required
- Optional
- Description
-
Parameter defines the chart that is used to retrieve the top charts and up to
500
results. Chart parameters could be found in store parsers. For instance:topselling_free
will retrieve the top free apps;topselling_paid
will retrieve the top paid apps.
Search Query
-
- Name
-
q
- Required
- Optional
- Description
-
Parameter defines the query you want to search. You can use any advanced search operators that you would use in a regular Google Play Store search (e.g.
pub:"SEGA"
).
Localization
-
- Name
-
gl
- Required
- Optional
- Description
-
The default parameter
US
defines the country of the store. Check the full list of supported Googlegl
countries.
-
- Name
-
hl
- Required
- Optional
- Description
-
The default parameter
en
defines the language of the store. Check the full list of supported Googlehl
languages.
Filters
-
- Name
-
store_device
- Required
- Optional
- Description
-
Default parameter
phone
- defines the store device of theapps
orgames
store. There is a list of available store devices:windows
,phone
,tablet
,tv
,chromebook
,watch
andcar
.
-
- Name
-
price
- Required
- Optional
- Description
-
Parameter defines the price filter that is used only together with
q
search parameter withapps
andbooks
stores. There are 2 options for a price filter:
1
will only display free items from given search query;
2
will only display paid items from given search query.
-
- Name
-
age
- Required
- Optional
- Description
-
The parameter is utilized to filter content based on the age of the target audience. It offers three distinct options:
age_range1
option targets content suitable for an audience up to 5 years old.age_range2
option targets content suitable for an audience aged between 6 and 8 years old.age_range3
option targets content suitable for an audience aged between 9 and 12 years old.
store=apps
andcategory=FAMILY
.store=movies
andcategory=FAMILY
.store=books
andcategory=coll_1689
.
Pagination
-
- Name
-
next_page_token
- Required
- Optional
- Description
-
Parameter defines the next page token that is used to retrieve the next page of results.
Engine
-
- Name
-
engine
- Required
- Required
- Description
-
Parameter defines an engine that will be used to retrieve real-time data. Current engine -
google_play_store
.
API Key
-
- Name
-
api_key
- Required
- Required
- Description
-
The
api_key
authenticates your requests. Use it as a query parameter (https://www.searchapi.io/api/v1/search?api_key=YOUR_API_KEY
) or in the Authorization header (Bearer YOUR_API_KEY
).
API Examples
Games Store - Landing page
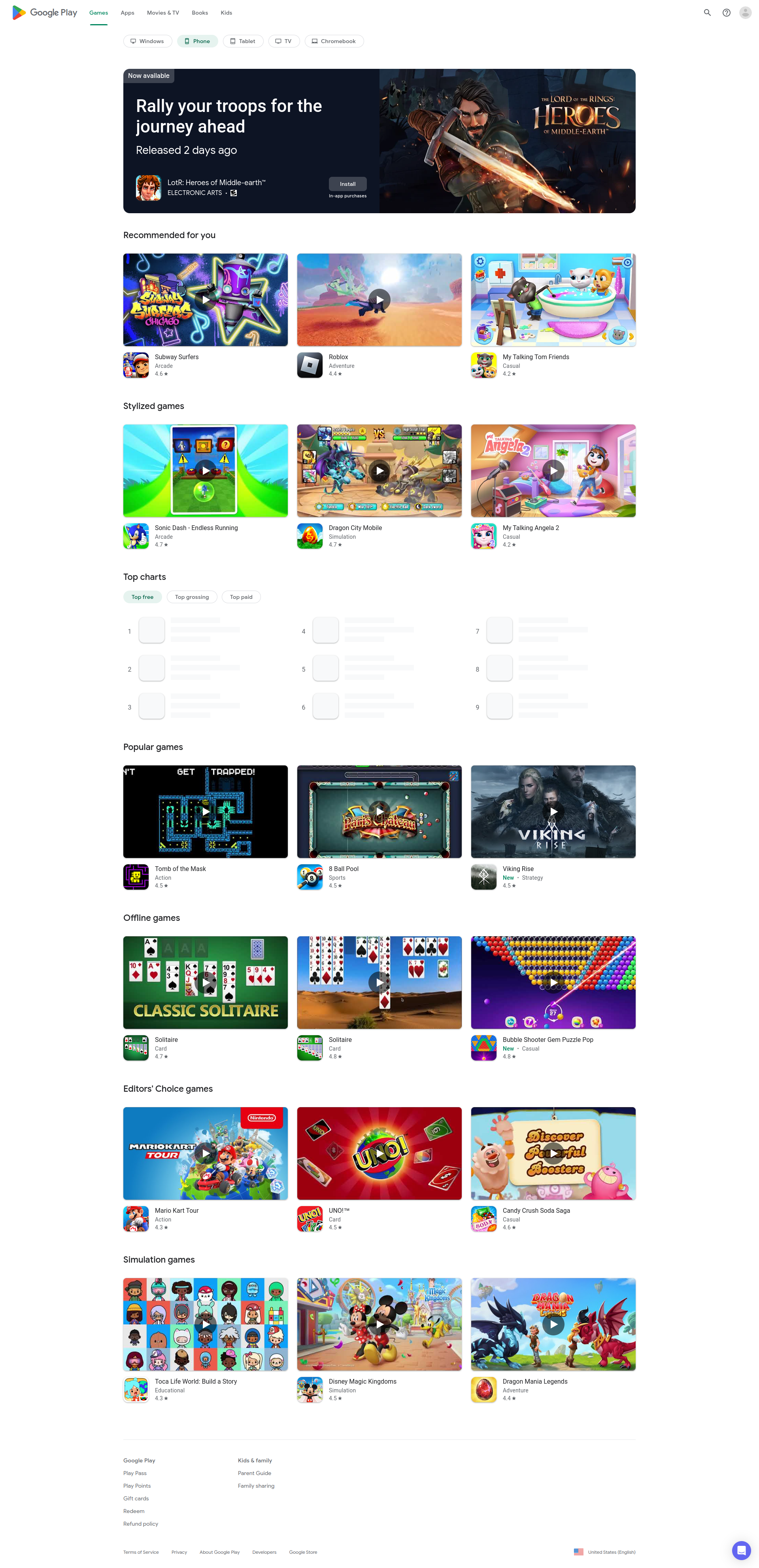
https://www.searchapi.io/api/v1/search?engine=google_play_store&store=games
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_play_store",
"store": "games"
}
response = requests.get(url, params=params)
print(response.text)
{
"top_charts": [
{
"title": "Top free",
"id": "topselling_free"
},
{
"title": "Top grossing",
"id": "topgrossing"
},
{
"title": "Top paid",
"id": "topselling_paid"
}
],
"organic_results": [
{
"title": "Recommended for you",
"items": [
{
"title": "Subway Surfers",
"link": "https://play.google.com/store/apps/details?id=com.kiloo.subwaysurf",
"product_id": "com.kiloo.subwaysurf",
"video": "https://www.youtube.com/embed/Zq74BVEoXSY?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Arcade",
"rating": 4.6,
"thumbnail": "https://play-lh.googleusercontent.com/IHpPJDyaTx3wZ6Bqk5RRI3d1SxDlq_hiR8UQ7A7XpZ3t7d4qehI0OTUkqp8wy3b6SQ=s64"
},
{
"title": "Roblox",
"link": "https://play.google.com/store/apps/details?id=com.roblox.client",
"product_id": "com.roblox.client",
"video": "https://www.youtube.com/embed/HsiMDb-mn5s?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Adventure",
"rating": 4.4,
"thumbnail": "https://play-lh.googleusercontent.com/WNWZaxi9RdJKe2GQM3vqXIAkk69mnIl4Cc8EyZcir2SKlVOxeUv9tZGfNTmNaLC717Ht=s64"
},
{
"title": "My Talking Tom Friends",
"link": "https://play.google.com/store/apps/details?id=com.outfit7.mytalkingtomfriends",
"product_id": "com.outfit7.mytalkingtomfriends",
"category": "Casual",
"rating": 4.2,
"thumbnail": "https://play-lh.googleusercontent.com/_Ub7v4j6_rVA26PX7KkAwCLaF6nfsrpwMfh1mfhukwp6FLNrc6vHqwn1CwffBR7PUuc=w416-h235"
},
{
"title": "Candy Crush Saga",
"link": "https://play.google.com/store/apps/details?id=com.king.candycrushsaga",
"product_id": "com.king.candycrushsaga",
"video": "https://www.youtube.com/embed/j11dUiYKUFs?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Casual",
"rating": 4.6,
"thumbnail": "https://play-lh.googleusercontent.com/TLUeelx8wcpEzf3hoqeLxPs3ai1tdGtAZTIFkNqy3gbDp1NPpNFTOzSFJDvZ9narFS0=s64"
},
{
"title": "Solitaire",
"link": "https://play.google.com/store/apps/details?id=com.smilerlee.klondike",
"product_id": "com.smilerlee.klondike",
"video": "https://www.youtube.com/embed/Ro0vwbKzbzI?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Card",
"rating": 4.7,
"thumbnail": "https://play-lh.googleusercontent.com/J3s5ht4Eu8UuVek-D4I5ArBX_4WB10wLH_mRdvq_Uku0wTimjCadg_TDhP1Y7JTVNuQ=s64"
},
{
"title": "My Talking Tom 2",
"link": "https://play.google.com/store/apps/details?id=com.outfit7.mytalkingtom2",
"product_id": "com.outfit7.mytalkingtom2",
"category": "Casual",
"rating": 4.2,
"thumbnail": "https://play-lh.googleusercontent.com/SBAkbpp2wn3HAKQm8A2s4s13-1rZzOIHoxS3Y_T_D7OAJx4q16YD8WxT7amLgncYwHs=w416-h235"
},
{
"title": "Sonic Dash - Endless Running",
"link": "https://play.google.com/store/apps/details?id=com.sega.sonicdash",
"product_id": "com.sega.sonicdash",
"video": "https://www.youtube.com/embed/XuCHvxzaysY?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Arcade",
"rating": 4.7,
"thumbnail": "https://play-lh.googleusercontent.com/4F-WwVKAs56rT6DGSfu1-9sW4MqSjenlIUqWS1K_8iB25ktsHKXXScAwJonvwo7DuMA=s64"
},
{
"title": "Solitaire - Classic Card Games",
"link": "https://play.google.com/store/apps/details?id=beetles.puzzle.solitaire",
"product_id": "beetles.puzzle.solitaire",
"category": "Card",
"rating": 4.7,
"thumbnail": "https://play-lh.googleusercontent.com/Rq9NMtmx2PAbiKP_vh1PzZ576aAVahIRJlQVlp-4NR1PAr9NXo4rbBM1AEcGWlEORu7Y=w416-h235"
},
{
"title": "Dragon City Mobile",
"link": "https://play.google.com/store/apps/details?id=es.socialpoint.DragonCity",
"product_id": "es.socialpoint.DragonCity",
"video": "https://www.youtube.com/embed/lKdd0lhS8eY?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Simulation",
"rating": 4.7,
"thumbnail": "https://play-lh.googleusercontent.com/ls7_gEbcVHb0QYLxApB_d8iymHiU6sp0EbZhh4lP2_jREAdpnVo3aor7eyyJSMDlZPw=s64"
},
{
"title": "My Talking Angela 2",
"link": "https://play.google.com/store/apps/details?id=com.outfit7.mytalkingangela2",
"product_id": "com.outfit7.mytalkingangela2",
"video": "https://www.youtube.com/embed/vN0erzakur4?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Casual",
"rating": 4.2,
"thumbnail": "https://play-lh.googleusercontent.com/nGQsSeJDngGdZ8Yt7h5j8lsTd9qekx_8Z5TuWJEkONO4fns_ESugndPQKpq63nDHq34=s64"
},
{
"title": "Solitaire",
"link": "https://play.google.com/store/apps/details?id=com.betterforsol.solitaire.card.games.free",
"product_id": "com.betterforsol.solitaire.card.games.free",
"video": "https://www.youtube.com/embed/WUNJVnSxkuw?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Card",
"rating": 4.8,
"thumbnail": "https://play-lh.googleusercontent.com/xst4n7LfIbey3HtFRncdLCwaig670p3d1Wy6EF2CmgSNlACrStjohgoPLpYDNaBUfg=s64"
},
{
"title": "Coloring Games: Color & Paint",
"link": "https://play.google.com/store/apps/details?id=com.rvappstudios.kids.coloring.book.color.painting",
"product_id": "com.rvappstudios.kids.coloring.book.color.painting",
"video": "https://www.youtube.com/embed/NAKctif7Nyo?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Educational",
"rating": 4.1,
"thumbnail": "https://play-lh.googleusercontent.com/zEeVdf2QNSOGP5b_koYGD72o_LB5uLdABUoBvYSxNiPZjVRwcxF2j2a_e8c5KGgpKxk=s64"
},
{
"title": "Mario Kart Tour",
"link": "https://play.google.com/store/apps/details?id=com.nintendo.zaka",
"product_id": "com.nintendo.zaka",
"video": "https://www.youtube.com/embed/ZwEalAfEbYw?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Action",
"rating": 4.3,
"thumbnail": "https://play-lh.googleusercontent.com/Nhdcc77MHYfXR9LoVhhkpnKbhwpZpCLKfl8dUwVhyqgflBQ5ROBtLsn_2fIongMYeoo6=s64"
}
]
},
{
"title": "Stylized games",
"items": [
{
"title": "Sonic Dash - Endless Running",
"link": "https://play.google.com/store/apps/details?id=com.sega.sonicdash",
"product_id": "com.sega.sonicdash",
"video": "https://www.youtube.com/embed/XuCHvxzaysY?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Arcade",
"rating": 4.7,
"thumbnail": "https://play-lh.googleusercontent.com/4F-WwVKAs56rT6DGSfu1-9sW4MqSjenlIUqWS1K_8iB25ktsHKXXScAwJonvwo7DuMA=s64"
},
{
"title": "Dragon City Mobile",
"link": "https://play.google.com/store/apps/details?id=es.socialpoint.DragonCity",
"product_id": "es.socialpoint.DragonCity",
"video": "https://www.youtube.com/embed/lKdd0lhS8eY?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Simulation",
"rating": 4.7,
"thumbnail": "https://play-lh.googleusercontent.com/ls7_gEbcVHb0QYLxApB_d8iymHiU6sp0EbZhh4lP2_jREAdpnVo3aor7eyyJSMDlZPw=s64"
},
{
"title": "My Talking Angela 2",
"link": "https://play.google.com/store/apps/details?id=com.outfit7.mytalkingangela2",
"product_id": "com.outfit7.mytalkingangela2",
"video": "https://www.youtube.com/embed/vN0erzakur4?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Casual",
"rating": 4.2,
"thumbnail": "https://play-lh.googleusercontent.com/nGQsSeJDngGdZ8Yt7h5j8lsTd9qekx_8Z5TuWJEkONO4fns_ESugndPQKpq63nDHq34=s64"
}
]
},
{
"title": "Popular games",
"items": [
{
"title": "Tomb of the Mask",
"link": "https://play.google.com/store/apps/details?id=com.playgendary.tom",
"product_id": "com.playgendary.tom",
"video": "https://www.youtube.com/embed/6MI2KYJwYyk?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Action",
"rating": 4.5,
"thumbnail": "https://play-lh.googleusercontent.com/V3M-ZVyu4NCOR8oxeHEQSrt5LJhu44xlDtIx-d3YnrPCMEvV-lxPJDyWoJkxsS1Soxsx=s64"
},
{
"title": "8 Ball Pool",
"link": "https://play.google.com/store/apps/details?id=com.miniclip.eightballpool",
"product_id": "com.miniclip.eightballpool",
"video": "https://www.youtube.com/embed/MkKWx0uVyRw?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Sports",
"rating": 4.5,
"thumbnail": "https://play-lh.googleusercontent.com/bPz1guJ6FHF3oIOEy3KqwpaDDKO-hLRaZoyzmM8bLFLN8fWm6L0_EuUnkwv9iqPo3Ag=s64"
},
{
"title": "Viking Rise",
"link": "https://play.google.com/store/apps/details?id=com.igg.android.vikingriseglobal",
"product_id": "com.igg.android.vikingriseglobal",
"video": "https://www.youtube.com/embed/XiebqruMwGk?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Strategy",
"rating": 4.5,
"thumbnail": "https://play-lh.googleusercontent.com/_7jYxlA0BbP8xFKW3tNJGrL0V27PTDNxjOHE5H0w1ScvsNbnkpUvUC9k0xflbUmt0G0=s64"
},
{
"title": "Magic Tiles 3",
"link": "https://play.google.com/store/apps/details?id=com.youmusic.magictiles",
"product_id": "com.youmusic.magictiles",
"video": "https://www.youtube.com/embed/-2zDjI0X04M?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Music",
"rating": 3.9,
"thumbnail": "https://play-lh.googleusercontent.com/roGkhpU3GfmHjgGr3xfbyEInDmUNYZloBgLdfA9gOCAnlOejqWozqPUaEY26nGlzVxE=s64"
},
{
"title": "Coin Master",
"link": "https://play.google.com/store/apps/details?id=com.moonactive.coinmaster",
"product_id": "com.moonactive.coinmaster",
"video": "https://www.youtube.com/embed/STPiRTKOlyA?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Casual",
"rating": 4.6,
"thumbnail": "https://play-lh.googleusercontent.com/Gpp87vMy7HMftEnl8GkTJrWPf2g6lIbNR0asWPtFBNSduXxfdzuky2fK1itlx4pfjaU=s64"
}
]
},
{
"title": "Offline games",
"items": [
{
"title": "Solitaire",
"link": "https://play.google.com/store/apps/details?id=com.smilerlee.klondike",
"product_id": "com.smilerlee.klondike",
"video": "https://www.youtube.com/embed/Ro0vwbKzbzI?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Card",
"rating": 4.7,
"thumbnail": "https://play-lh.googleusercontent.com/J3s5ht4Eu8UuVek-D4I5ArBX_4WB10wLH_mRdvq_Uku0wTimjCadg_TDhP1Y7JTVNuQ=s64"
},
{
"title": "Solitaire",
"link": "https://play.google.com/store/apps/details?id=com.betterforsol.solitaire.card.games.free",
"product_id": "com.betterforsol.solitaire.card.games.free",
"video": "https://www.youtube.com/embed/WUNJVnSxkuw?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Card",
"rating": 4.8,
"thumbnail": "https://play-lh.googleusercontent.com/xst4n7LfIbey3HtFRncdLCwaig670p3d1Wy6EF2CmgSNlACrStjohgoPLpYDNaBUfg=s64"
},
{
"title": "Bubble Shooter Gem Puzzle Pop",
"link": "https://play.google.com/store/apps/details?id=com.pop.bubbleshooter.gem.classic.games",
"product_id": "com.pop.bubbleshooter.gem.classic.games",
"video": "https://www.youtube.com/embed/GlsupufPe8w?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"category": "Casual",
"rating": 4.8,
"thumbnail": "https://play-lh.googleusercontent.com/RdGSE0y044g8WvhzQViktRnL48s32i9ibl9d8XuLqLwW1nnX4m_qtQo5kXDAXJ5-gQ=s64"
}
]
}
],
"highlights": [
{
"product": {
"title": "LotR: Heroes of Middle-earthâ„¢",
"author": "ELECTRONIC ARTS",
"cta": "Install",
"cta_snippet": "In-app purchases"
},
"id": "com.ea.gp.capitalphx",
"feature": "Now available",
"title": "Rally your troops for the journey ahead",
"subtitle": "Released 2 days ago",
"link": "https://play.google.com/store/apps/details?id=com.ea.gp.capitalphx",
"thumbnail": "https://play-lh.googleusercontent.com/1iZy-jkmU9Utcfe__80Vf0zjrFYvia0dB-roH2xa8ziPbfLavc7b7Fe6sRedUL2JdZah6TA9XOEK=w648-h364"
},
{
"id": "mc_editorialmd_nintendo_games_fcp",
"feature": "Trending",
"title": "The best Nintendo games for on-the-go fun",
"subtitle": "Mario, Pikmin & more",
"link": "https://play.google.com/store/apps/editorial?id=mc_editorialmd_nintendo_games_fcp",
"thumbnail": "https://play-lh.googleusercontent.com/5TWA1qLfvIphLaoiOjkRRAgrLN9UKMBb7ziPrVQa6zTa7pc5L7HCPSwp64tFv3uN3iz2FnU_w20=w648-h364"
}
],
"pagination": {
"next_page_token": "EAgawgEICBDv0-rpBxDgyJavDxD86Kn2BxDg-tChARDL873zAhDuqqitDBCDgr_OBxCI1q33AhiysZOCCRiPodPrBRjN_pzZDxiqyPqLDRjp8PTABRiwzo5dGOOIt88MGPC094MNGK_XhcYKGK3EwbkLGMW8_tcLGIjPk5wDGOXX6fMJGKr6mp0BGIDfxOcLGPv36PsGGPiL7IQDGPP8xNAGGJ6QqlkYypu_sQoqGHZNa0JYQ2RWNVE5WkZzUWIzSzNPNnc9PQ"
}
}
Apps Store - Action games category
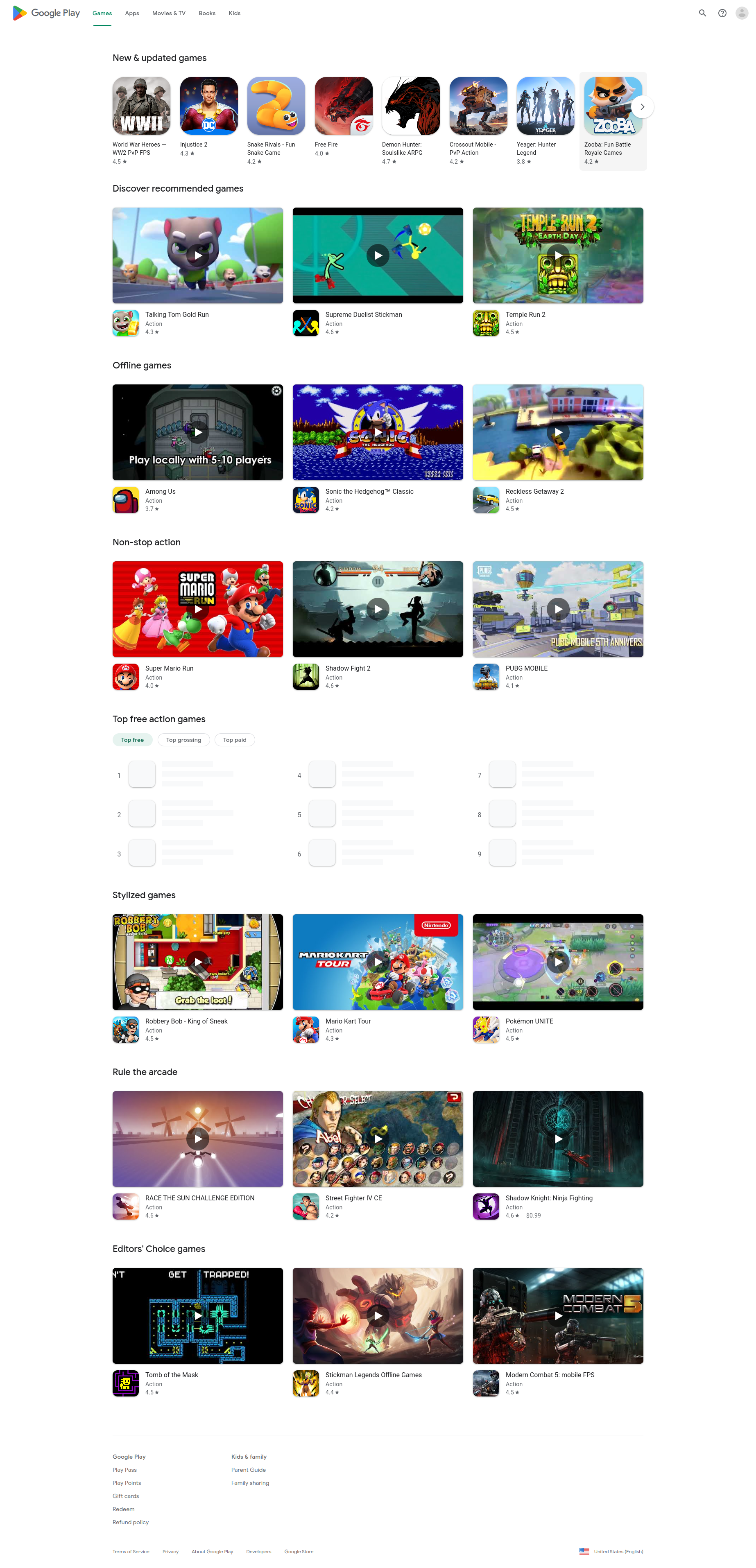
https://www.searchapi.io/api/v1/search?category=GAME_ACTION&engine=google_play_store&store=apps
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_play_store",
"store": "apps",
"category": "GAME_ACTION"
}
response = requests.get(url, params=params)
print(response.text)
{
"top_charts": [
{
"title": "Top free",
"id": "topselling_free"
},
{
"title": "Top grossing",
"id": "topgrossing"
},
{
"title": "Top paid",
"id": "topselling_paid"
}
],
"organic_results": [
{
"title": "New & updated games",
"items": [
{
"title": "World War Heroes — WW2 PvP FPS",
"product_id": "com.gamedevltd.wwh",
"link": "https://play.google.com/store/apps/details?id=com.gamedevltd.wwh",
"rating": 4.5,
"thumbnail": "https://play-lh.googleusercontent.com/hK5_ZNBujg7orY0Hqlg4J7x2MeV7sI8wWStlwhiM2Afio7dQuFJfouFmhoFxb62ebqyS=s256"
},
{
"title": "Injustice 2",
"product_id": "com.wb.goog.injustice.brawler2017",
"link": "https://play.google.com/store/apps/details?id=com.wb.goog.injustice.brawler2017",
"rating": 4.3,
"thumbnail": "https://play-lh.googleusercontent.com/qSXDMLN9q1qo-Rd7PXg_shmKR4S9PN_A-wsFUdngL8y8sy_fBfjrB-befd63W2-C8jo=s256"
},
{
"title": "Snake Rivals - Fun Snake Game",
"product_id": "com.supersolid.snake",
"link": "https://play.google.com/store/apps/details?id=com.supersolid.snake",
"rating": 4.2,
"thumbnail": "https://play-lh.googleusercontent.com/zukordpVpskgVoQbJpTjTdYwRljPKjiqHKXsvUFJElkdBtJNbHiA3WItm-swJASdQCs=s256"
},
{
"title": "Free Fire",
"product_id": "com.dts.freefireth",
"link": "https://play.google.com/store/apps/details?id=com.dts.freefireth",
"rating": 4.0,
"thumbnail": "https://play-lh.googleusercontent.com/WWcssdzTZvx7Fc84lfMpVuyMXg83_PwrfpgSBd0IID_IuupsYVYJ34S9R2_5x57gHQ=s256"
},
{
"title": "Demon Hunter: Soulslike ARPG",
"product_id": "com.eapublishing.dhsw.free",
"link": "https://play.google.com/store/apps/details?id=com.eapublishing.dhsw.free",
"rating": 4.7,
"thumbnail": "https://play-lh.googleusercontent.com/soSBgfM68dJ0DDWgcvZvhrgOlz3sGI_lf2kHBeGtf4utwQw0OUuPmRy0h63_v6cd6EQ=s256"
}
]
},
{
"title": "Discover recommended games",
"items": [
{
"title": "Talking Tom Gold Run",
"product_id": "com.outfit7.talkingtomgoldrun",
"link": "https://play.google.com/store/apps/details?id=com.outfit7.talkingtomgoldrun",
"category": "Action",
"rating": 4.3,
"video": "https://www.youtube.com/embed/7ZTo9Gpwzws?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"thumbnail": "https://play-lh.googleusercontent.com/gXHJLOPxbvDkx6pD8VYxwDpqiOsfQKq-kJOCdRiaBdmX0CaAARy_WV4fJjQKT7KG0uI=s64"
}
]
}
],
"pagination": {
"next_page_token": "EAgaxAEICBDgyJavDxD07aLjBRDuqqitDBDL873zAhC6l9GNDRD86Kn2BxCHh8WaBhDI1YKTBxjHz7jdCBi33s2bDBjTm4S8Axj79-j7Bhj14OjCBhiP_eqXCRiPvZ_WDhiYhvm7Dxiule3JARik48n5DhjwtPeDDRixgLi7BBjAtYDfBRiF7bjjDRjTqsG8Ahjxhv7cCRivucjsCBiP0b7YDBjM3pTyBhiQoczUBCoYcDBOcmFzSjM0K0JMek95VWE2N21zZz09"
}
}
Top Charts - Best selling paid apps in US
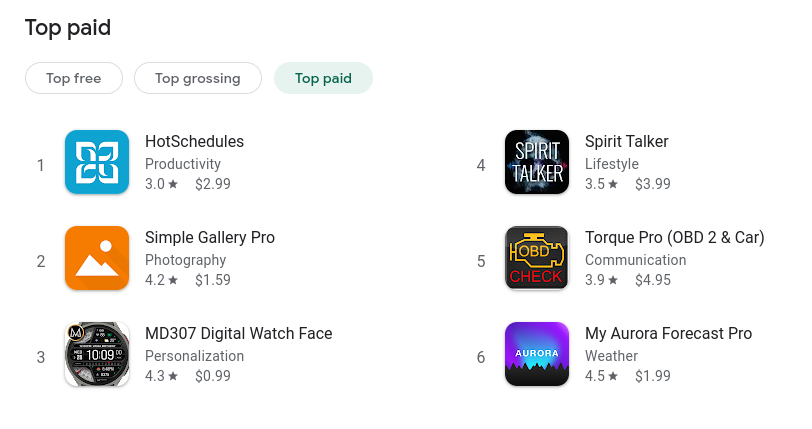
https://www.searchapi.io/api/v1/search?chart=topselling_paid&engine=google_play_store&store=apps
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_play_store",
"store": "apps",
"chart": "topselling_paid"
}
response = requests.get(url, params=params)
print(response.text)
{
"top_charts": [
{
"title": "HotSchedules",
"product_id": "com.tdr3.hs.android",
"link": "https://play.google.com/store/apps/details?id=com.tdr3.hs.android",
"rating": 3.0,
"author": "HotSchedules",
"category": "Productivity",
"description": "​HotSchedules is the industry's leading employee scheduling app because it’s the fastest and easiest way to manage your schedule and communicate with your team. Team members love it because they can swap, pick-up or release shifts with one click. Work-life balance is easily managed with automatic shift pick ups when you want more hours and time off requests for when you don’t. Calendar sync and notifications keep your Schedules and the roster automatically updated to your phone with any manager-approved changes.Managers appreciate the 75% time savings when building schedules and one-click approvals for shift changes. The ability to monitor business performance with sales and labor snapshots from anywhere, keeps them out of the back office. And most importantly, staying connected with broadcast and one-to-one messaging makes for a happy and productive team culture. NOTE: The HotSchedules application REQUIRES a valid HotSchedules user account through your employer. Need help? Contact Customer Care 24x7x365",
"price": "$2.99",
"extracted_price": 2.99,
"currency": "USD",
"thumbnail": "https://play-lh.googleusercontent.com/cudBRDzsvi50wundWmjnvvaotvejOERDJP4XDur6VtmeiI3aBdGEYmqNVc3Knev5BA",
"images": [
"https://play-lh.googleusercontent.com/fy5RAtDgfH3r_3PD-0cMeafq1MIF067IEeoVGOqoC1zh36edFxxYQBJtiuV7hGQTCEke",
"https://play-lh.googleusercontent.com/pbMcNtSCDQH9bzWH2Ck0_3d2g2GG2IE3Jfpm1NBR2gha2y6xHThpYpELSTS6Hs5pBgo",
"https://play-lh.googleusercontent.com/cvOzyHqL7t014h7Tzq5fqlKymktWuBphf9NLJPoG-XV30jBoAGPHY6OxtkGFVlGgFpM",
"https://play-lh.googleusercontent.com/sJo6nISnOqO76BfgAil4PvBi12ZaynkYyq5ukw_KQfV5V8QX9gCzlXbZqzUUgsCWBc0",
"https://play-lh.googleusercontent.com/hI202JBUVQXZsmg-NIa_t37oCGu7chbEZvyQ_ZBYlw9nqrXxfJ0VzyNTxziflvDjffM",
"https://play-lh.googleusercontent.com/y4iWKL9i1oYt2Pgsf-UfFdK1sEV1NNBqvhlWQAZcCK1L25W_Z70D2ItzpwuEmKgoHg",
"https://play-lh.googleusercontent.com/ygatQQxwalam0kQs3Pr7GhlfLUisj8xo0IHfQhzFCkwh2SfKRubW_fPYpGS_UhTIaVrI"
]
},
{
"title": "Simple Gallery Pro",
"product_id": "com.simplemobiletools.gallery.pro",
"link": "https://play.google.com/store/apps/details?id=com.simplemobiletools.gallery.pro",
"rating": 4.2,
"author": "Simple Mobile Tools",
"category": "Photography",
"description": "Simple Gallery brings you all the photo viewing and editing features you have been missing on your Android in one stylish easy-to-use app. Browse, manage, crop and edit photos or videos faster than ever, recover accidentally deleted files or create hidden galleries for your most precious images and videos. And with advanced file-support and full customization, finally, your gallery works just the way you want.ADVANCED PHOTO EDITORTurn photo editing into child's play with Simple Gallery's improved file organizer and photo album. Intuitive gestures make it super easy to edit your images on the fly. Crop, flip, rotate and resize pictures or apply stylish filters to make them pop in an instant.ALL THE FILES YOU NEEDSimple Gallery supports a huge variety of different file types including JPEG, PNG, MP4, MKV, RAW, SVG, GIF, Panoramic photos, videos and many more, so you enjoy full flexibility in your choice of format. Ever wonder \"Can I use this format on my Android\"? Now the answer is yes.MAKE IT YOURSSimple Gallery's highly customizable design allows you make the photo app look, feel and work just the way you want it to. From the UI to the function buttons on the bottom toolbar, Simple Gallery gives you the creative freedom you need in a gallery app.RECOVER DELETED PHOTOS & VIDEOSNever worry about accidentally deleting that one precious photo or video you just can't replace. Simple Gallery allows you to quickly recover any deleted photo and videos, meaning on top of being the best media gallery for Android, Simple Gallery doubles as an amazing photo vault app.PROTECT YOUR PRIVATE PHOTOS, VIDEOS & FILESRest assured your photo album is safe. With Simple Gallery's superior security features you can use a pin, pattern or your device’s fingerprint scanner to limit who can view or edit selected photos and videos or access important files. You can even protect the app itself or place locks on specific functions of the file organizer.It comes with material design and dark theme by default, provides great user experience for easy usage. The lack of internet access gives you more privacy, security and stability than other apps.Contains no ads or unnecessary permissions. It is fully opensource, provides customizable colors.Check out the full suite of Simple Tools here:https://www.simplemobiletools.comFacebook:https://www.facebook.com/simplemobiletoolsReddit:https://www.reddit.com/r/SimpleMobileToolsTelegram:https://t.me/SimpleMobileTools",
"price": "$1.59",
"extracted_price": 1.59,
"currency": "USD",
"video": "https://play.google.com/video/lava/web/player/yt:movie:QF16dkirH5o?autoplay=1&embed=play",
"thumbnail": "https://play-lh.googleusercontent.com/uaTwQIlEjPHHsqdpnmxLoT_XAgFtLXCYFncGAc85xs0hoEnYqLiANRwjnQXzgLcDHxs",
"images": [
"https://play-lh.googleusercontent.com/cmLIESBIlKc1LKX1HYhPCXSrp90AZjjsvUzgnqcoKXKKHC3kMyhs4ru1jydmUTondrw",
"https://play-lh.googleusercontent.com/OYsP1KUCxj2ONKzHljvFykO73JXeCSiA1om5NZiVAfLpEyKEMy7aY_1Vmq8WOHqCK2s",
"https://play-lh.googleusercontent.com/TWWjQYhG7uLD6dfMcS0jYODcEum4DnZPJMuQmC95tN9yvSyWpzHSzdJfZt6mYXFCm30",
"https://play-lh.googleusercontent.com/H2gkVkKflK6eTVxu-aUsXv-D5r_i5Ch_o4ls79hZZLzl9tenecozO8bu-p0ljdbtEuBP",
"https://play-lh.googleusercontent.com/qNZZk7nORHMQ0S9hDJF_zpXeFeAd0TkFsHyGEaFSeZ3bfTtR6OFWJAtYp2KoW16GpYM",
"https://play-lh.googleusercontent.com/HNjzv6Mcft0UX0iZUOxoRR7JdqSJrDpDWd1MnQWez1Q11_czb2Lyd165hCgHrs9iCA",
"https://play-lh.googleusercontent.com/15LiW-wLE9aEgFvlkQKY3rHe80mkd9CIl34-1ffR1OzpiyWWnAG7w7YNf_il2DHLGg",
"https://play-lh.googleusercontent.com/0h6PuRLczz_oLxp6MM0qyg2bZJB1i0rfebF9e56PaxXlfTnTBDENDrjFcLklMC36tfnG",
"https://play-lh.googleusercontent.com/YLzCHhq6TS85tt8BlRkZHRd6wXCeJYWiPhsE3cOIuVa8Vyh-xIRs2f_SoZ8p9zblnbk",
"https://play-lh.googleusercontent.com/UlEGJpqMuLhL2gFTGrLXU9AGXxo18lMeTgyIydSljruhgnZ-gGBpqQbZgwgIGu3asQ8N",
"https://play-lh.googleusercontent.com/KlpOXWP-1bpeiX_gfMaAM4sa3xd6JuSU5LEnpymlo0pQcPh0NlPEe2Xn9zB6IeDdlN3x"
]
},
{
"title": "MD307 Digital Watch Face",
"product_id": "com.watchfacestudio.md307digital",
"link": "https://play.google.com/store/apps/details?id=com.watchfacestudio.md307digital",
"rating": 4.3,
"author": "Matteo Dini MD ® Watch Faces",
"category": "Personalization",
"description": "The MD307 is a Modern Digital watch face for Wear OS.It contains 3 Preset App shortcuts, 1 customizable shortcut, moon phase, steps, daily goals, heart rate + intervals*, 3 customizable complications where you can have the data you prefer such as weather (etc), changeable colors and more.WATCH FACE INSTALLATION NOTES:Please check this link for installation and troubleshooting guide:https://www.matteodinimd.com/watchface-installation/This watch face supports all Wear OS devices with API Level 28+ like Samsung Galaxy Watch 4, Galaxy Watch 5, Pixel Watch etc.Watch face features:- 12/24hr Digital Time- Date - Moon phase- Battery- Heart rate + Intervals*- Steps- Daily steps goal set to 8500 st/day- 3 Preset app shortcuts- 1 customizable shortcut- 3 customizable complications- Always ON Display supported with changeable colors and minimal style- Changeable colors of time, date, day, seconds, foreground, bars and general colorsWatch face customization:1 - Touch and hold display2 - Tap on customize optionWatch face preset APP Shortcuts:- Calendar- Battery- Measure HRWatch face complications:you can customize with the any data you want.For example, you can select weather, time zone, sunset/sunrise, barometer etc.*Heart Rate Notes:The watch face does not automatically measure and does not automatically display the HR result when installed.To view your current heart rate data you’ll need to take a manual measurement. To do this, tap on the heart rate display area. Wait a few seconds. The watch face will take a measurement and display the current result.Make sure you have allowed the use of the sensors when installed the watchface otherwise swap with another watch face and then come back to this to enable the sensors. .After the first manual measurement, the watch face can automatically measure your heart rate every 10 minutes. Manual measurement will also be possible.**some features may not be available on some watches.Let's keep in touch !Matteo Dini MD ® is a well-known and ultra-awarded brand in the watch faces world!Some references:Best of Galaxy Store Awards 2019 Winner:https://developer.samsung.com/sdp/blog/en-us/2020/05/26/best-of-galaxy-store-awards-2019-winner-matteo-dini-on-building-a-successful-brandSamsung mobile press:https://www.samsungmobilepress.com/feature-stories/samsung-celebrates-best-of-galaxy-store-awards-at-sdc-2019Matteo Dini MD ® is also a registered trademark in the United States and Europe.Newsletter:Sign up to stay updated with new watch faces and promotions!http://eepurl.com/hlRcvfFACEBOOK:https://www.facebook.com/matteodiniwatchfacesINSTAGRAM:https://www.instagram.com/mdwatchfaces/TELEGRAM:https://t.me/mdwatchfacesWEB:https://www.matteodinimd.com-Thank you !",
"price": "$0.99",
"extracted_price": 0.99,
"currency": "USD",
"thumbnail": "https://play-lh.googleusercontent.com/2K6X6jm-kh91QgPIeBpUO6IIqZ_ugNcjJw7F1nZKY0s6z2PjWtzE3s9cfHr5OW_XjQ",
"images": [
"https://play-lh.googleusercontent.com/b-pVzOKnlIQ5zZwVqIngstZCYbHQ8mq0xXxntdvo4fZ6zcsKzmXFuMKpsSFkb6vFrAkE",
"https://play-lh.googleusercontent.com/-W8lVBlBPFpjPs47TgYHpQ5M67CqtkEGqYcfUAhC9eRwtuKiRbbKnJ3BQkXOYBCprxM",
"https://play-lh.googleusercontent.com/ZmsHYC2crr_AcLGFXk3Jlms3VD4YhBpmX-3-v1PamVQCDqRsivWjMDs3NXCUEPk8w10",
"https://play-lh.googleusercontent.com/RLqfRCzVrYFmzp5AmmF_fwzAx2GfZ6uJ56MP_NtHCKEvas7L0LW_WuSrmjqO522bBKHx",
"https://play-lh.googleusercontent.com/L4_W8FlnPhe8R3UHYn5aSZiXuRWjZvQsTYZeK0WSc4xgwaHw1bzUWLPUSPteWgmk7SVf",
"https://play-lh.googleusercontent.com/iwbwlbIkgMGOegXqe06MRKsCNn3jXECMaP3VDvswxar2mZrFaDIzscIk08BvtYZnHA",
"https://play-lh.googleusercontent.com/zn8aTVDijK5ZI55l3ltmVvoRYC2YAonwwAMvt_nVbtKWFp4WdvdgHBuS_qA8Id7OEhSM",
"https://play-lh.googleusercontent.com/fYX_PuHXoasZ9PCf1aEBjXh-9lTzFZm5xU7OOLdSQ-vWKvVdKyTZw7jlaF94Q5kPa60",
"https://play-lh.googleusercontent.com/LhxHXfVKJ2ymaxKsdvWDH28VGI1UL5TXU3sqGTT_dY9aF17sMo3O7SCN-8ocOveNuw",
"https://play-lh.googleusercontent.com/7SOwZ2wu8sK3tp2lmcYrzz3viZkQQ5XrV2jEadyUi9vvLYffV4mSLsQAn0AbJ0jUKg4p",
"https://play-lh.googleusercontent.com/qg2qG8CzQMtx8EumeJKTQCdEsMk4oBu5A0ZdxsPaZEfqp31y722cu2jXWeE9efyi65s",
"https://play-lh.googleusercontent.com/qz01MSvgxsoMDcP0ixcgO9eQcb-F9vz0KcPPDLdovzoMl6I1HeHxzjJDtMhf-jVkkaM",
"https://play-lh.googleusercontent.com/zBT6ZpKMJahiTW5Lzrlxue4mkNuDlaQJ4GkemAwRmskkFzunOY8KqGnFLHs0Tw9SPgeH",
"https://play-lh.googleusercontent.com/b6toGB6IW6aiXEgA_fxY55yXJ66U41O8y6YKUbabfjjOTtOHSL3VDZ21Pjzq_fbEI5ES",
"https://play-lh.googleusercontent.com/QwCRyGkfGdCgKbPSgWVwi6ECb6hXYBEarNCVe9qM3nZ7VdmdVEBUCgwuzjfoNQnVKl0",
"https://play-lh.googleusercontent.com/0hcAV2qsyAgZLBO_UnA0x3FeRI7lE1pJUO1GbwKVBAW2UlQ2l7d7fuSwAcGFMe6L1eU",
"https://play-lh.googleusercontent.com/1Wyo1JkbOSJYyYtRp4KSziM2-S96Vs02a93txATTJDsbwAyZbRShaBRE368fnvO3d8o",
"https://play-lh.googleusercontent.com/L5hqRhpc4lKKOb2u-5xgA8qA9PvxM8iqYnj-c-k_jlnhHSJ-HxWQAjRxgKU6d8jWTHqi",
"https://play-lh.googleusercontent.com/ak6BUzTznv6Ix36X0Uymt6SJ6Smp7FoYFpmeTrk88c9L8F2ivD8wHyRkYgvsJp2mlRw",
"https://play-lh.googleusercontent.com/juL8JFuIOMrWwyJMR1WW-Jmrdt-iF9EZk9tfO5979k183mRCAUlmatf_-sBpt-oTs8w",
"https://play-lh.googleusercontent.com/1cbpnvxUUHvG8DA6L6YTdnbP3AcWl_Koq1r1EONJ5t-1G7xB7kIlZFtxAOFUd2V-MQ",
"https://play-lh.googleusercontent.com/e7kul9LGD22M4JdKF626EH0CD-Jx3Gj0VxNCsZoI_jiNyzT5p1Ur10e65ThV-sIJ7-oI",
"https://play-lh.googleusercontent.com/6leV8LBg-u0mri0hB-yXjNbcgNOrMEmTF9DLnerQ1RWVSNDvjwXAfxPuE02Csqqthb4I",
"https://play-lh.googleusercontent.com/LwBYs9sPejZwkhahngrhdGTzhkoLYrv1CwZMuPWmq1fw0sVPqEPr3wZUZ9TK_OSHKA",
"https://play-lh.googleusercontent.com/gtylYbjk_PSXwsXPnRdQof0E7bk5VqKZW8n8UtYIOX2XdIkb7Vls0fqmc7lUeJ3BrIY",
"https://play-lh.googleusercontent.com/Lp-FtIC3msb5SoRrKCjgfjf0g0G6G9_juA2oca2iNuQqdRcbGASljLrKmhzBxLj29Pw",
"https://play-lh.googleusercontent.com/Jxo6WArXyhnWFE0GzW9__tUthpmyi8fSk6NtgrxUyuu9g0tFgutN2n6zhFmgeKT6pjs",
"https://play-lh.googleusercontent.com/SQht5280z8x_ZH2ZZ0NtkOHGh8BCC8CFcuue6fsGZ35gFvL7yZNkc7v0Xn123a79z9kv",
"https://play-lh.googleusercontent.com/ee1UCWeqyKleMbm4skf5rHLPqjZfSxkZnESqWNz3xrTUExHnbN00LLtXGD1KW06Cn2U",
"https://play-lh.googleusercontent.com/er6Tj9PudT2dEV-WNbtQDkE0_PumiTeRVa80ltJr5FKB-S6wXUiFojQnbkzSfStQJTE",
"https://play-lh.googleusercontent.com/9Rd9vMkfME94mqPbb16YwZEt_LYnKTSJZwk_jMl5CfW719654WuMaU0-G-xWBSL4Eu0E",
"https://play-lh.googleusercontent.com/LmBQXkjLoJrRaqkRfJgmOk0WrHTibh2j0p5ADsisSlPBMFi7JrV1DH4JbEUKj3rGIc0",
"https://play-lh.googleusercontent.com/nNulYzHp3jR06q1atXw2V1G4Qjm2Ufg6pQ7RC2SCIhvIdFU7jTY3L03F_Q9OK_yvVpxm",
"https://play-lh.googleusercontent.com/PioSfryQzhSCNqPHbdWmV0WGXaw-USwa7bq0LM2cI9lHBYtV9O72xkCdcn6gDrA0tg",
"https://play-lh.googleusercontent.com/yEJOfYeZOLjndTivVJU0f4EfHmnM_Rv82HaGFhKFAD5Jk7f1tpXzN7da8LGxxhNtvoI",
"https://play-lh.googleusercontent.com/haGaChC3zAlBd2rMmbZzY5QoRF6i5kf9zDj9Y96eu3qHg6ufAlnsHfy30NTwLHdAOQ",
"https://play-lh.googleusercontent.com/HEfeV-HfFPFEjzcCadu5nf_lXxQ77N82xWSc-ukOh73zbUj-RY9vMssQNqMwSGUcPw",
"https://play-lh.googleusercontent.com/EAMIWfmzCf-l9us9CfdFSFFgf5-bkLNwcLOGiy1yjek7Zr8w2BiAhPgZT8O2q6pQ_6bw",
"https://play-lh.googleusercontent.com/p7PA_-IXIfM9KKoPapNwedA7nM-F3JqHB3ILOIHQaqaERp20WTuEPNebb7pm7r5XlQ",
"https://play-lh.googleusercontent.com/P8zkHRwtS-a7ObAo1cACGtsWuLlOqNrSUB6md0E7I4oqEiN1LaBXHz8SrMGHht040dw5"
]
},
{
"title": "Spirit Talker",
"product_id": "com.SpottedGhosts.SpiritTalker",
"link": "https://play.google.com/store/apps/details?id=com.SpottedGhosts.SpiritTalker",
"rating": 3.5,
"author": "Spotted: Ghosts",
"category": "Lifestyle",
"description": "The Spirit Talker works in a similar way to the world famous Ovilus device.It produces words and speech based on what the sensors in your phone are detecting.The theory behind this app is that spirits might be able to manipulate the device sensors to say and show relevant words and responses to questions being asked during a paranormal investigation. The Spirit Talker is a modern form of ITC (Instrumental Trans Communication) and is very simple to use. Just click start and begin asking your questions.When a response is detected by the app the words will show visually in the text box along with audible speech. When you have finished your session just click stop.You can also look back at the responses you received during your session by clicking on the folder button (this only works when the scanner is \"Stopped\").You can turn the EMF meter on/off by pressing the Magnet button.You can enter the settings area by clicking the Cog button. To get back to a previous screen either click the Back button or the Cog button.The sensors that the app uses are:Magnetometer (EMF)AccelerometerGyroscopeGravityHumidityTemperatureAir pressureThe EMF Meter only works if your device has a Magnetometer Sensor. If not then the EMF Meter won't be displayed. Please check the compatibility of your phone / tablet.When you have finished your session, turn off the scanner to save the words to the file.*******************************This app uses the Google Text to Speech engine in order to speak the wordsIf your device is not speaking the words, please check to make sure your device has got the Google Text to Speech app installed. If it is not installed, please install it from here:https://play.google.com/store/apps/details?id=com.google.android.tts&hl=en_GB&gl=USAlso, if the voice sounds robotic then it probably means that you have not got the Google Text to Speech engine installed and the app is using a default voice synthesiser that is built into your device. If so, please download the Google Text to Speech app on the the link above.You can change the device voice by going to \"Settings\" -> \"Accessibility\" -> \"Text-to-speech output\"********************************* Disclaimer **Use at your own risk. We cannot be held personally responsible for you or any outcome (paranormal or otherwise) from using this app!The paranormal is not a proven science and is considered theoretical. Accordingly, words or phrases generated are not intended as requests or instructions, and should not be used to make legal, financial, medical or other important decisions. Words/phrases generated do not represent the official position of the developer.Refer to our website for a full list of terms and conditions http://www.spirittalkerapp.com/",
"price": "$3.99",
"extracted_price": 3.99,
"currency": "USD",
"thumbnail": "https://play-lh.googleusercontent.com/CVGRudYVa8CVU2WrYTXWMLQjZbNCXnhyybhcKD4L7VcR_BgA_ZrblvLMgsvDsdLi48Q",
"images": [
"https://play-lh.googleusercontent.com/1DEkYdvrMkThKda35vYiHCJCeMbtky6x2T5eTyD9mAroYWJwIq9fRruBNCYQYGHdpmrX",
"https://play-lh.googleusercontent.com/E1pFc-tf-oOF_YQusU6clIvvt4gXbDYeH8w5c2y3AOOY4de8oXLHSKfm_w6NKHX3oN1U",
"https://play-lh.googleusercontent.com/uNhRhO-d2XIzMRI7yVR0p2nlZGqSNGaRZw7CHSiw3S4Y-hj1ks8W4MtfCc7-k2iFKA",
"https://play-lh.googleusercontent.com/bxFOUntRyXEbVlrRWhojwF9v3fyKHEWfGL-OIRrx-zaR19BfyqibsFe1Z-65E5oQsQ",
"https://play-lh.googleusercontent.com/35hf3MH-UMjwRWjV0YFWfTn2Vn2FEsjq_TNnCd6BAfYIfyRG2j4p9axDO6ARIAvn",
"https://play-lh.googleusercontent.com/FeRjGfn-uoWI-zAPCE6e7XL8UcK3oU2EqID0qIwh1QqLD1DfPKo-sHKMzTUgkowDsCE",
"https://play-lh.googleusercontent.com/qb_bROj5wsfnAzc40ydty1srKAIZAiaDchIl2Q1tP_iIQQwSbwVFTFe0uiMNrAZjVzg",
"https://play-lh.googleusercontent.com/Ziy55YwjLI6Tgm02VFCo6Lri4idAFFzqbYHBEC8_AnP2AC1QcNeLwvRRVp9Re9pvglqC",
"https://play-lh.googleusercontent.com/ouuzX0iVYaMbmnTBLUtC9ka8gVjuPVL1yiPER5VOspW8vraEVbPIkz9fOq9dsd2zhXQ",
"https://play-lh.googleusercontent.com/HIzmWqM8v_emGvqNl1bBEf4bfJBwMFWkQEaJgLFju6ffLUmk9royMY2XfbR01nFDbQ",
"https://play-lh.googleusercontent.com/PtwaGlshXLeoQTSbtvsoKWvd2Nm4F-_LR3W8IEb-87swrPBU_x5lIaH7DnWntO5h9DM",
"https://play-lh.googleusercontent.com/Gm1gEw2Qey1gWfNYcuQCQ7JPKfMvWZKf0EqpUsx72PittykgCNRZOtkp1Pam3dlTN-A"
]
},
{
"title": "Torque Pro (OBD 2 & Car)",
"product_id": "org.prowl.torque",
"link": "https://play.google.com/store/apps/details?id=org.prowl.torque",
"rating": 3.9,
"author": "Ian Hawkins",
"category": "Communication",
"description": "See what your car is doing in realtime, get OBD fault codes, car performance, sensor data and more!Torque is a vehicle / car performance / diagnostics tool and scanner that uses an OBD II Bluetooth adapter to connect to your OBD2 engine management / ECULayout your own dashboard with the widgets / gauges you want!It can use the GPS to provide tracker logs with OBD engine logging so you can see what you were doing at any point in timeIt can also show and reset a DTC / CEL / fault code like a scantool. Helps you fix your car and helps keep repair costs down!Torque also features:* Dyno / Dynomometer and Horsepower/HP & Torque* Can read Transmission Temperatures (vehicle dependant)* 0-60 speed timings - more accurate than just using plain old GPS - see how fast your car is (or truck )* CO2 emissions readout* Customisable dashboard & profiles* Video your journey using the Track Recorder plugin with onscreen OBDII data overlay - a black box for your car/truck!* Automatically send GPS tagged tweets directly to twitter (for example if you are going on a road trip)* Massive fault code database for lookup of fault codes from different manufacturers* Theme support (choose from different themes to change the look of your dashboard)* Send logging information to web or email CSV/KML for analysis via excel / openoffice reader* Heads up display / HUD mode for night time driving* Compass (GPS Based) that won't suffer magnetic interference* GPS Speedometer/Tracking and realtime web upload capability - see what you were doing and your engine, at a point in time* Turbo boost feature for vehicles that support MAP and MAF sensors (VW & Golf / Audi / Seat etc supported)* Alarms and warnings (for example if your coolant temperature goes over 120C whilst driving) with voice/speech overlay* Car dock support* Graph data* MPG* Able to share screenshots to Facebook, Twitter, Google+, Email, etc* AIDL API for third party apps, A simple Telnet interface for developers to talk to the adapter, and an OBD scanner.* Works on tablet devices like the Motorola Xoom, Dell Streak, Samsung Galaxy Tab and NookWorks on any vehicle that uses the OBD 2 standard (most vehicles built after 2000, but can work for vehicles as far back as 1996) if in doubt check with your manufacturer first or look for 'OBD2' on written on a big white label in your engine bayWorks on vehicles made by Ford, VW, GM/Vauxhall/Opel, Chrysler, Mercedes, Volkswagen, Audi, Jaguar, Citroen, Peugoet, Skoda, Kia, Mazda, Lexus, Subaru, Renault, Mitsubishi, Nissan, Honda, Hyundai, BMW, Toyota, Seat, Dodge, Jeep, Pontiac and many more vehicle makes, European, US, Far East, etc. Some vehicle ECUs may support more/less features than othersThe app needs a Bluetooth OBD2 adapter to work. The adapter is small and plugs into the diagnostics socket on the car which gives your phone access. You can find a list of adapters on: http://torque-bhp.com/wiki/Bluetooth_Adapters (Garmin EcoRoute HD adapters not supported)If you buy one of the cheap china OBD2 ELM327 bluetooth adapters from ebay / amazon, then make sure you have a good returns policy with the seller Some of the best reliable OBD2 / OBD adapters are the Scantool.net adapter, the OBDKey, and PLX Devices, OBDLink, Bluetooth, ELM327 and other adapters are supported - search on google for themMore features added every release - there are forums at http://torque-bhp.com/forums/ - if you want something added then let me know and I'll try to add it if it is possible! Vehicle ECUs vary in the amount of sensors supported*PLEASE NOTE* Any reports of hanging or reboots after quitting the app is due to a bug in HTC devices and Galaxy Tab,this can be triggered by using the Pandora/Vlingo/Other BT apps. Disabling Pandoras new bluetooth settings fixes the problem, but HTC/Samsung need to release a fix.Please contact their support and request a fix,Thanks!Changelog: http://torque-bhp.com/forums/?wpforumaction=viewtopic&t=3.0",
"price": "$4.95",
"extracted_price": 4.95,
"currency": "USD",
"video": "https://play.google.com/video/lava/web/player/yt:movie:I3PmFyYYgsk?autoplay=1&embed=play",
"thumbnail": "https://play-lh.googleusercontent.com/jCmUBkfai1vJhnCYXDefs706hLCrBAPtzDX4JI4aFuVza2MS-ZMSSJnJFSFEHciDU9s",
"images": [
"https://play-lh.googleusercontent.com/Rwna0Z6-EYHkQb18SaFfCRpk1mfsLE3uC0UQMx_dxf48biXeD3QFsNJrnJjutWMFmh8",
"https://play-lh.googleusercontent.com/IElU7AcmrAvI8yDW1YEPVrDfxuizWKSLkUtWyIDaZScE2jwlDvxyKG2z2PD5yu1jEg",
"https://play-lh.googleusercontent.com/cRIYvWimJPcTcVvlpR47XskhrAtLQwZKKUeZEYqbdhe1tI91F8EsFceLcLJFGNTx1J3x",
"https://play-lh.googleusercontent.com/w7VRn1Mi-jIE1CxjQUADpvbylag34xSzvMeMGwqzYXAr4sVndiZhIredfufvXoRRu_yu",
"https://play-lh.googleusercontent.com/Hkd4cH3xiiCGXddoS27UAB_lTLO2FwYFPz35j7ZA96TMi8y3kC3c-5kVNZwyLNYrZ5k",
"https://play-lh.googleusercontent.com/gbyACckmx2FB8wzK-7__8nX91y2I7lEH2-PDp4p4Pgegnuex5Bqx4q1fJbGt5A-Cigg",
"https://play-lh.googleusercontent.com/uhLsPMZ5BIQwSrtC0BAkNxwTNmWQX4t-oZdvbmWAb5hG4Q7oi69-jA492Q8ZK8gieA",
"https://play-lh.googleusercontent.com/ps72wQBmIOJbFdADS88rs0ubrkK5ESOpBfOb6tinePsypUHtsAL8NfugPTOVVUsSyLU",
"https://play-lh.googleusercontent.com/bu0bkX6u5NdvFmmk89Vef-kTGm6PLM6wSijtTPYmtBrEgSwpzSdLiRgwhZUpwkmKTJV0",
"https://play-lh.googleusercontent.com/XQHptNoUSlAvQseylASvA8CaYFdvO_o9dHaX8sb3l88RHo7NlRwOcNCO4KAZgPO1RyCd",
"https://play-lh.googleusercontent.com/_sySIsbM6IH5YFvo03LierCgOGRbG21K98VdLBKt7I1_lVCSRD6tHv2Qapg7uHwsxuA",
"https://play-lh.googleusercontent.com/gBU6JNmZSAKZKnzTPtHGgCBCJKetN20XJ3-U4QCXCaCGNlvWtRpxjNU9Mbm08844eCd6",
"https://play-lh.googleusercontent.com/kO7aAjPZ_NOa-deANkeoRUkng0orMhy2yb8fknVBuWhnjEGVte5UX-AQ0oObJVt6Bw",
"https://play-lh.googleusercontent.com/82wikNpCVUKD1MiZrDT-fAbJBN0FtyTebRE3Ho9mYqQmBzF-WR706F7a8q7PRmtP8w",
"https://play-lh.googleusercontent.com/iS-Sod_krWXgkqBmvFuu4ooMW_uskNsjz3UQ3LoS9JdfbHMPQhmy3oRsFV2ecI_sOpg",
"https://play-lh.googleusercontent.com/cJ1v1Nlch3DX_1UQsky_LF7vUF4zWc11JwSAKkipu3N0a4WI1GJTFu2LwVbJJM3xqdk",
"https://play-lh.googleusercontent.com/cbzzoJAkP3442EXf_749ZIRhYxs0I-hLUCwxThbL3WwXEw5agnJ12AOKAtvFkV2lx0QT"
]
},
{
"title": "My Aurora Forecast Pro",
"product_id": "com.jrustonapps.myauroraforecastpro",
"link": "https://play.google.com/store/apps/details?id=com.jrustonapps.myauroraforecastpro",
"rating": 4.5,
"author": "jRustonApps B.V.",
"category": "Weather",
"description": "My Aurora Forecast Pro is the best app for seeing the Northern Lights. Built with a sleek dark design, it appeals to both tourists and serious aurora watchers by telling you what you want to know - whether that is exactly how likely you are to see the aurora borealis or details about the solar winds and high-resolution sun imagery. With this app, you'll be seeing the Northern Lights in no time.- Find the current KP index and how likely you are to see the Northern Lights.- View a list of the best locations to view from right now.- Map showing how strong the aurora is around the world, based on the SWPC ovation aurora forecast.- Push notifications and alerts when auroral activity is expected to be high.- Forecasts for the next hour, several hours and several weeks so you can plan your Northern Lights viewing long in advance (subject to weather conditions).- Solar wind statistics and sun imagery.- View live aurora webcams from around the world.- Tour information so if you're considering to go to locations such as Iceland or even Alaska or Canada, you'll be able to find tours that we can recommend to you.- Pro version offers the same great functionality of My Aurora Forecast but without any advertisements!If you want the latest updates on geomagnetic activity and enjoy viewing the aurora borealis, this app is right for you.",
"price": "$1.99",
"extracted_price": 1.99,
"currency": "USD",
"thumbnail": "https://play-lh.googleusercontent.com/LinDbGFwe9aPAjPNFhr4NexlagSe30coHBsZQKo9PgFt0ZUNJ9fgmnYDcnMbjlFdPJY",
"images": [
"https://play-lh.googleusercontent.com/xtQVNypmdofKyEDiQo6gzYCPH_U-AGYcj6wr5EOI9bUDottaWtILbnSu8vhfLUlh400",
"https://play-lh.googleusercontent.com/0siSZh8oUjAyooZboXZ4VWCLfDnJq39eOijeoiMd0LwyUJZWmDjSlAMjnrtNG9Qm3PB7",
"https://play-lh.googleusercontent.com/Zf3JIMvyC8FhDY_4QcUttScz5D2UYCHgvgPgyiflKYQ1eTuKDAzQ2ictgWLGTuufig",
"https://play-lh.googleusercontent.com/T9DjMOKrQzlzEGn5wW3YUa9OG4bKJ1ovLztdrqXBa2nFDtK6eBJVpiiLPPy0urEHxAc",
"https://play-lh.googleusercontent.com/PLVBmNVE329k1CbD9OPclc6wq0m4rUKDhu6jz6zZlxQV4FLk3sbvrVCnRLfdREiuEXq5",
"https://play-lh.googleusercontent.com/FWtRYLVYOmpCk4K-uvnnit_lTuvyNAjAqcsu43LOgkRi94n5jWoemH7H4DfKE8jsOaM",
"https://play-lh.googleusercontent.com/Yj-wPyIKNN5v1x9cWi1smEUZY1XUltujjxF7ZM-U5mGVU6scbO2qPkx2OnAMQp__s34",
"https://play-lh.googleusercontent.com/Oe9_mD7YLN_ljSTCx8oqnoSLaLzLK-Oc-cucS2SDqCmD7-J-z2ZAUX-DqwIMuBp1Pw",
"https://play-lh.googleusercontent.com/3jhyhHlW9s77O5oqhWtV9J58YUaUEtlh1eWZewbEl1IZ0kdI8mBlRL1id6grS5jkGQ",
"https://play-lh.googleusercontent.com/A0qvp5X930XQF2CRoRks74wRxg0mJ0pBZz1qPLfQ6eaGtFPG8k5FH7gLRakOgNeLwW4"
]
}
]
}
Games Store - Tablet device
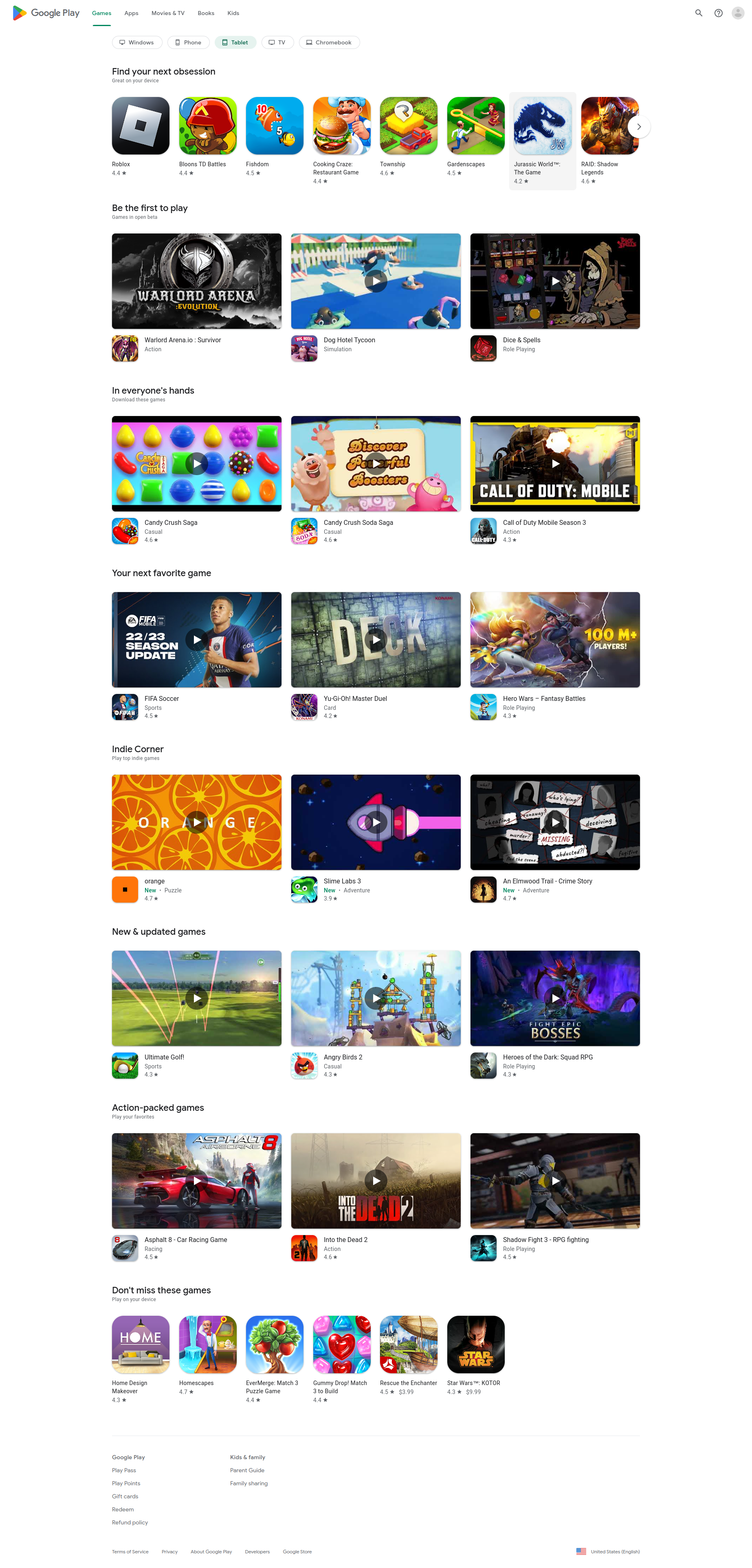
https://www.searchapi.io/api/v1/search?engine=google_play_store&store=games&store_device=tablet
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_play_store",
"store": "games",
"store_device": "tablet"
}
response = requests.get(url, params=params)
print(response.text)
{
"organic_results": [
{
"title": "Find your next obsession",
"subtitle": "Great on your device",
"items": [
{
"title": "Roblox",
"link": "https://play.google.com/store/apps/details?id=com.roblox.client",
"product_id": "com.roblox.client",
"rating": 4.4,
"thumbnail": "https://play-lh.googleusercontent.com/WNWZaxi9RdJKe2GQM3vqXIAkk69mnIl4Cc8EyZcir2SKlVOxeUv9tZGfNTmNaLC717Ht=s256-rw"
},
{
"title": "Bloons TD Battles",
"link": "https://play.google.com/store/apps/details?id=com.ninjakiwi.bloonstdbattles",
"product_id": "com.ninjakiwi.bloonstdbattles",
"rating": 4.4,
"thumbnail": "https://play-lh.googleusercontent.com/LeZ9qnLV9Sc8dM0nzQrKPoEsrOiT5mpEYFIs91ztvcq3NWZNJBA2e0U7UsG4WNRxyeA=s256-rw"
},
{
"title": "Fishdom",
"link": "https://play.google.com/store/apps/details?id=com.playrix.fishdomdd.gplay",
"product_id": "com.playrix.fishdomdd.gplay",
"rating": 4.5,
"thumbnail": "https://play-lh.googleusercontent.com/8drwwDDkmYeyjE_h1EctwjzPjVkoLoGwn1wY0a9pYaeX18GzwuM9dH5801Qyridli3g=s256-rw"
},
{
"title": "Cooking Craze: Restaurant Game",
"link": "https://play.google.com/store/apps/details?id=com.bigfishgames.cookingcrazegooglef2p",
"product_id": "com.bigfishgames.cookingcrazegooglef2p",
"rating": 4.4,
"thumbnail": "https://play-lh.googleusercontent.com/QCyn1CV8e97NOf0l0MnYpZz3N1n5RDgEIMs5LBJnLd6Nl2lyPxwbymT4SqKjhKujeU0d=s256-rw"
}
]
},
{
"title": "Be the first to play",
"subtitle": "Games in open beta",
"items": [
{
"title": "Warlord Arena.io : Survivor",
"link": "https://play.google.com/store/apps/details?id=com.dreamplay.evoW.google",
"product_id": "com.dreamplay.evoW.google",
"category": "Action",
"thumbnail": "https://play-lh.googleusercontent.com/ThWFWiVw00nMKoczujEZpnijOV0xwl854_NQQuPlHgkoM44L8oyRWyXt4P3KMUdmUSg=w416-h235-rw"
},
{
"title": "Dog Hotel Tycoon",
"link": "https://play.google.com/store/apps/details?id=com.idle.dog.hotel.tycoon",
"product_id": "com.idle.dog.hotel.tycoon",
"video": "https://play.google.com/video/lava/web/player/yt:movie:_GzwVKBRvZo?autoplay=1&embed=play",
"category": "Simulation",
"thumbnail": "https://play-lh.googleusercontent.com/_8s5d84jbNrdmx9X0ZzcE0urngQE0uRMw2idSJ5dTA5SC78qUyW_rMh017yHaqIcnrGW=s64-rw"
}
]
}
],
"pagination": {
"next_page_token": "EAgaTAgIEKmlgtcCEKKI3M0HEJqs0p8HENC7odYGENWXtP4JEK_F2sICEOzrv6QBEOyk8OsIKhg4RGpndzJwYXZNTHBkVWFzNlNMNkdRPT0"
}
}
Movies Store - Family movies for kids under the age of 5
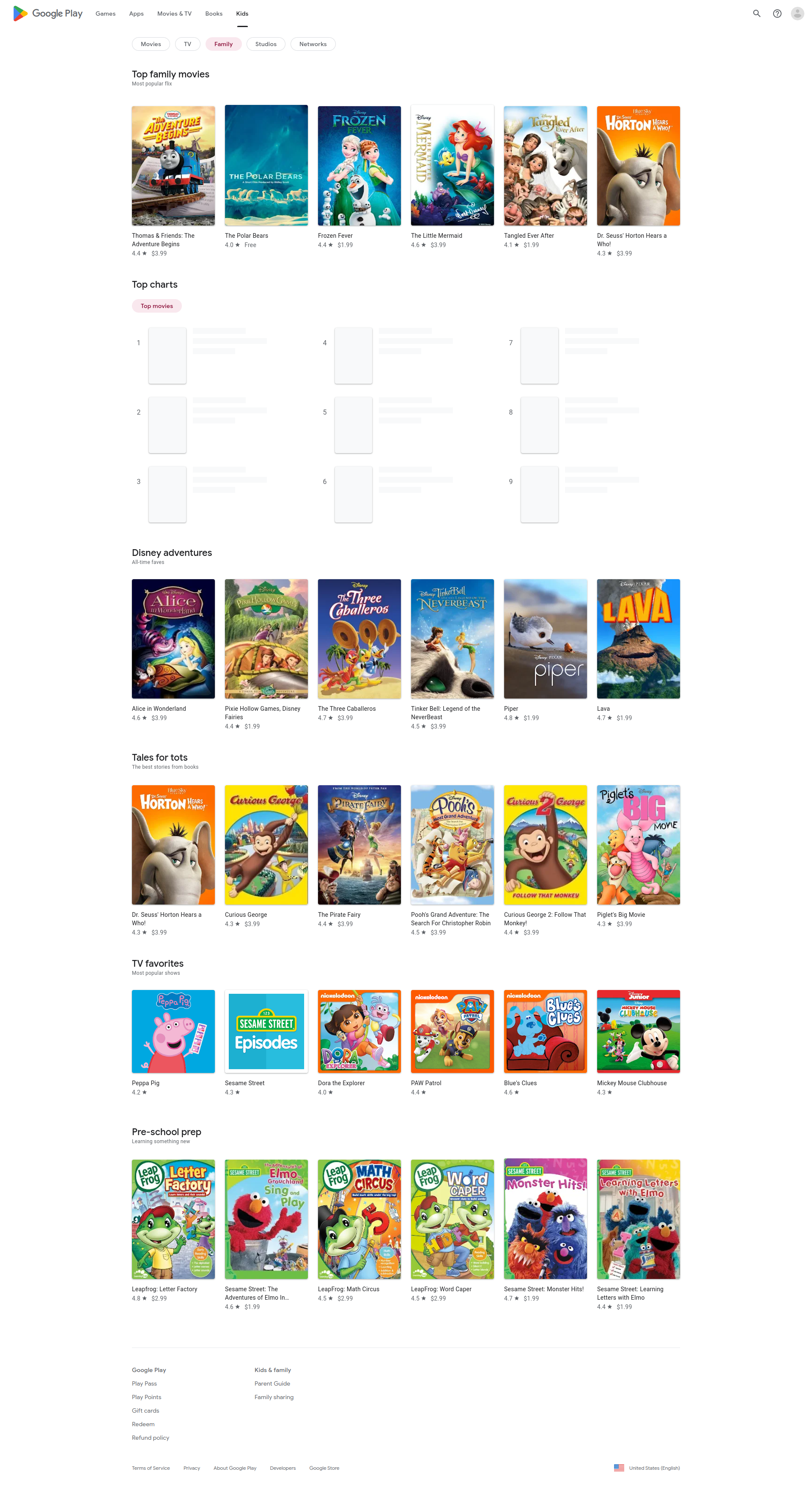
https://www.searchapi.io/api/v1/search?age=age_range1&category=FAMILY&engine=google_play_store&store=movies
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_play_store",
"store": "movies",
"category": "FAMILY",
"age": "age_range1"
}
response = requests.get(url, params=params)
print(response.text)
{
"top_charts": [
{
"title": "Top movies",
"id": "topselling_paid"
}
],
"organic_results": [
{
"title": "Top family movies",
"subtitle": "Most popular flix",
"items": [
{
"title": "Thomas & Friends: The Adventure Begins",
"link": "https://play.google.com/store/movies/details/Thomas_Friends_The_Adventure_Begins?id=ZbNbLO1nJ_w",
"product_id": "ZbNbLO1nJ_w",
"video": "https://play.google.com/video/lava/web/player/yt:movie:bPRgcqh5PnI?autoplay=1&embed=play",
"rating": 4.4,
"price": "$3.99",
"extracted_price": 3.99,
"thumbnail": "https://play-lh.googleusercontent.com/uoO6YPzZryf4fyLdFZ4qHPjx6p3eFbMSglLXtkNnXnrmBxO0sptes50SE_ZKnOBKOMF2Wg=s256-rw"
},
{
"title": "The Polar Bears",
"link": "https://play.google.com/store/movies/details/The_Polar_Bears?id=GBZ0KC2sG5Y",
"product_id": "GBZ0KC2sG5Y",
"rating": 4.0,
"price": "Free",
"thumbnail": "https://play-lh.googleusercontent.com/twKRv12Qtqxc7ko4fIQ9c3s_PYY3mRTYJC_sNk9a41rPN1F15SqzXLTOf5BODcG5Zv19=s256-rw"
},
{
"title": "Frozen Fever",
"link": "https://play.google.com/store/movies/details/Frozen_Fever?id=j2JOgXq2ANc",
"product_id": "j2JOgXq2ANc",
"video": "https://play.google.com/video/lava/web/player/yt:movie:5-ZH-pt8c40?autoplay=1&embed=play",
"rating": 4.4,
"price": "$1.99",
"extracted_price": 1.99,
"thumbnail": "https://play-lh.googleusercontent.com/8dfTF5mRG8jSmKUbjVPnm6bR4bnQUTkX0muZG31p55y9EZfBbTMXHqRAatfuiaimhVyZ=s256-rw"
}
]
}
],
"pagination": {
"next_page_token": "CiWq5rbCAx8ICBDYl-mQAxDb78vvDxC_1qvQDRCKkPUUEObQ2oYBEAY"
}
}
Movies Store - Search query
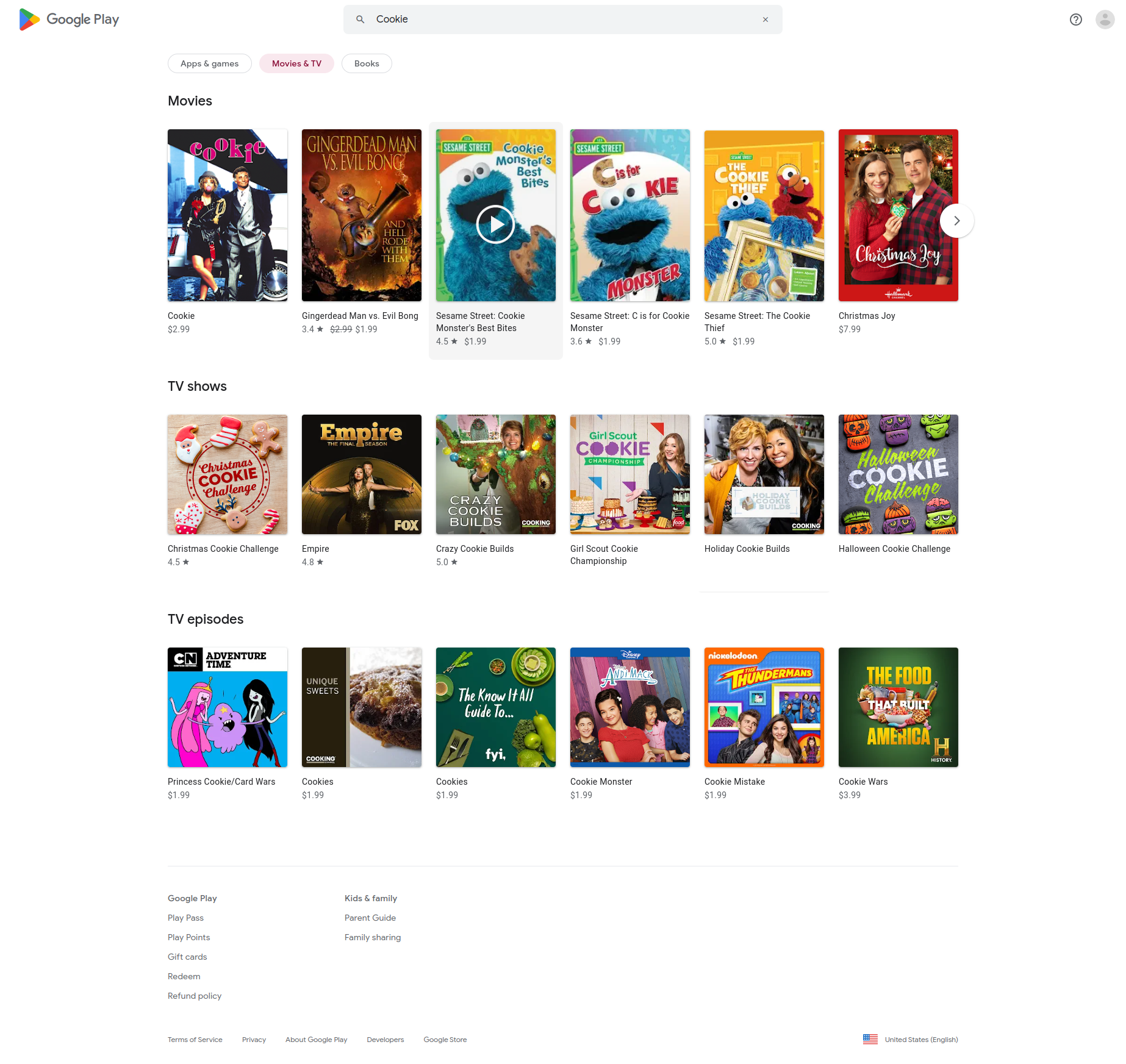
https://www.searchapi.io/api/v1/search?engine=google_play_store&q=Cookie&store=movies
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_play_store",
"store": "movies",
"q": "Cookie"
}
response = requests.get(url, params=params)
print(response.text)
{
"organic_results": [
{
"title": "Movies",
"items": [
{
"title": "Cookie",
"link": "https://play.google.com/store/movies/details/Cookie?id=Upvzti0CyM8.P",
"product_id": "Upvzti0CyM8.P",
"price": "$2.99",
"extracted_price": 2.99,
"thumbnail": "https://play-lh.googleusercontent.com/4MFPdGzzZxMQsUQC0rytzD4Kw9pqSMCWX8ymZe8FGT3myxdjCwMUghaJSSn7VM4W5kSzkcyAKth3ywXcUw8=s256"
},
{
"title": "Gingerdead Man vs. Evil Bong",
"link": "https://play.google.com/store/movies/details/Gingerdead_Man_vs_Evil_Bong?id=JsQnB0jGxho",
"product_id": "JsQnB0jGxho",
"video": "https://www.youtube.com/embed/c-1dYD53Nv4?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"rating": 3.4,
"original_price": "$2.99",
"extracted_original_price": 2.99,
"price": "$1.99",
"extracted_price": 1.99,
"thumbnail": "https://play-lh.googleusercontent.com/obGmUG0tSeqzG2My-w4G4At8xtFXMah5NhAEMKl_tbI2pEbGEjKpQVI3uYyM3baOmmkX=s256"
},
{
"title": "Sesame Street: Cookie Monster's Best Bites",
"link": "https://play.google.com/store/movies/details/Sesame_Street_Cookie_Monster_s_Best_Bites?id=ZAijlp_2454",
"product_id": "ZAijlp_2454",
"video": "https://www.youtube.com/embed/ntya81qspI0?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"rating": 4.5,
"price": "$1.99",
"extracted_price": 1.99,
"thumbnail": "https://play-lh.googleusercontent.com/xSmHzNSevQbSOhlCHXOXAvoLEsasq5DE6mgd6ejy8ye_MZbDToDqcq6bZthbvOMYi10=s256"
},
{
"title": "Sesame Street: C is for Cookie Monster",
"link": "https://play.google.com/store/movies/details/Sesame_Street_C_is_for_Cookie_Monster?id=T8m_fQFx9H8",
"product_id": "T8m_fQFx9H8",
"video": "https://www.youtube.com/embed/bZB3pTrZOco?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"rating": 3.6,
"price": "$1.99",
"extracted_price": 1.99,
"thumbnail": "https://play-lh.googleusercontent.com/ynwxRz2T7980OFHa-c0cnR8NLs1pvplK70DMJDDHDrHhvC80_xW84FccZx-Mg-QhGx6D=s256"
},
{
"title": "Sesame Street: The Cookie Thief",
"link": "https://play.google.com/store/movies/details/Sesame_Street_The_Cookie_Thief?id=C2S1PIb5wQE",
"product_id": "C2S1PIb5wQE",
"video": "https://www.youtube.com/embed/14HhR06KlaE?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"rating": 5.0,
"price": "$1.99",
"extracted_price": 1.99,
"thumbnail": "https://play-lh.googleusercontent.com/siingfatTIE22qN76UboLMWGxGr6nInjyd5cVnyyEREW0LXRXJSL3OtijYm_JVHeYAPhrQ=s256"
},
{
"title": "Christmas Joy",
"link": "https://play.google.com/store/movies/details/Christmas_Joy?id=5RjT7KEYWNk.P",
"product_id": "5RjT7KEYWNk.P",
"video": "https://play.google.com/video/lava/web/player/yt:movie:XFszBPR5E7k.P?autoplay=1&embed=play",
"price": "$7.99",
"extracted_price": 7.99,
"thumbnail": "https://play-lh.googleusercontent.com/J1YO1iQgTw6X6gI4H6wYu10VTYeBvwKQuuu8iZ1A3YBRl_orB942Kqp4Tr6cQHv_AzCbh8Kq-wbpbmKN5Q=s256"
},
{
"title": "Cookie",
"link": "https://play.google.com/store/movies/details/Cookie?id=0E1948AA6E2DF6A9MV",
"product_id": "0E1948AA6E2DF6A9MV",
"thumbnail": "https://play-lh.googleusercontent.com/proxy/8Lc_wo_gMiBq64wSt1CV8taAvMKV_IPvrQFyV3F-8isBr2Rxspj8uOpoqZosbvfI60aCN_zLpc2kiS_7xZ0tc9AiZVJkki1i8j2clXbkyyss8IfK3lhWMK2INLs_xTLYsMrhVuSe2K05LFk1C4ebmr6qTPAnh_MinCf3qA=s256"
},
{
"title": "Sesame Street: Elmo and Cookie Monster Supersized Fun",
"link": "https://play.google.com/store/movies/details/Sesame_Street_Elmo_and_Cookie_Monster_Supersized_F?id=WLmBUXTrM2g",
"product_id": "WLmBUXTrM2g",
"video": "https://www.youtube.com/embed/JoqD_B-2X1c?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"rating": 4.5,
"price": "$1.99",
"extracted_price": 1.99,
"thumbnail": "https://play-lh.googleusercontent.com/CVvjo03ZorEP1BblH41K6sFmVfCEK_PkhBu4OXnmsS9ybUpQmulWwJiAxNUlj4p1WlE=s256"
},
{
"title": "Sesame Street’s 50th Anniversary Celebration",
"link": "https://play.google.com/store/movies/details/Sesame_Street_s_50th_Anniversary_Celebration?id=-v6msJ1BpZQ.P",
"product_id": "-v6msJ1BpZQ.P",
"video": "https://play.google.com/video/lava/web/player/yt:movie:DTeIz4jKuvg.P?autoplay=1&embed=play",
"rating": 5.0,
"price": "$2.99",
"extracted_price": 2.99,
"thumbnail": "https://play-lh.googleusercontent.com/lAR4B_heHcRR841j_IZXpJm1vjPz-EXxob8E_ajQOV6U8mrjCF7XsE_qzaekM3mSN1pm5M2TpO88FKaFFYY=s256"
},
{
"title": "Alice in Wonderland",
"link": "https://play.google.com/store/movies/details/Alice_in_Wonderland?id=aBBL8axydx0",
"product_id": "aBBL8axydx0",
"video": "https://www.youtube.com/embed/kt4oPazlNlE?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"rating": 4.6,
"price": "$3.99",
"extracted_price": 3.99,
"thumbnail": "https://play-lh.googleusercontent.com/kdnI5f-9TYbNH-HdaPjO39yS3mWB0G4ULJgnLX3ElY1TT8DoVZsEjh54QSRQdN58fgEt=s256"
},
{
"title": "Small Time Crooks",
"link": "https://play.google.com/store/movies/details/Small_Time_Crooks?id=H20Ecyt1BIw.P",
"product_id": "H20Ecyt1BIw.P",
"video": "https://play.google.com/video/lava/web/player/yt:movie:9Ffs5uZMiPE.P?autoplay=1&embed=play",
"price": "$3.99",
"extracted_price": 3.99,
"thumbnail": "https://play-lh.googleusercontent.com/yuQYZdIC21ggag6rAFbyfYxyMoCG3KUjQ7YJPC9tiqUkwK2lceG3gw9NC_VmwQB5Wao9Dhr3qJNkyElIwUXk=s256"
},
{
"title": "Ralph Breaks the Internet",
"link": "https://play.google.com/store/movies/details/Ralph_Breaks_the_Internet?id=HOjK_x5s9ZU.P",
"product_id": "HOjK_x5s9ZU.P",
"video": "https://play.google.com/video/lava/web/player/yt:movie:39PzPcMc98I.P?autoplay=1&embed=play",
"rating": 3.7,
"price": "$3.99",
"extracted_price": 3.99,
"thumbnail": "https://play-lh.googleusercontent.com/TcAcqJet1CjSHsPaAr9hzV72f84Z0EjAdItbknzQTvQufMnnHBW09RPfbEq_wYBXx2FGiW4Bus6xt5kxrYhE=s256"
},
{
"title": "Despicable Me",
"link": "https://play.google.com/store/movies/details/Despicable_Me?id=qfl6oQoVeS8.P",
"product_id": "qfl6oQoVeS8.P",
"video": "https://play.google.com/video/lava/web/player/yt:movie:M_clYuq9tJw.P?autoplay=1&embed=play",
"rating": 4.4,
"price": "$3.99",
"extracted_price": 3.99,
"thumbnail": "https://play-lh.googleusercontent.com/qPoRwePtAgg-yK7Dqg-BgUxzBN1_rbMxBZMcOmYKHeTonnNatiBd5hmvKOMxJMapXlqPD70w3tLsdmSLq5g=s256"
},
{
"title": "Fortune Cookie Prophecies",
"link": "https://play.google.com/store/movies/details/Fortune_Cookie_Prophecies?id=YUPgV8anFwc",
"product_id": "YUPgV8anFwc",
"video": "https://www.youtube.com/embed/jCrGYV1lrO0?ps=play&vq=large&rel=0&autohide=1&showinfo=0",
"rating": 3.7,
"price": "$3.99",
"extracted_price": 3.99,
"thumbnail": "https://play-lh.googleusercontent.com/cLQSQ019eHcskcw4GmvtfJyCcPjoS28_Kv66Eq65OWTVaRermClKpaNjXjlrKp0_QOs-KQ=s256"
},
{
"title": "Wreck-It Ralph",
"link": "https://play.google.com/store/movies/details/Wreck_It_Ralph?id=QqsGKcWsACA.P",
"product_id": "QqsGKcWsACA.P",
"video": "https://play.google.com/video/lava/web/player/yt:movie:byOZy3D_IU0.P?autoplay=1&embed=play",
"rating": 4.5,
"price": "$3.99",
"extracted_price": 3.99,
"thumbnail": "https://play-lh.googleusercontent.com/Ns25JYAqmH8pSqDYe2lFR4xRL5nlE8ijfEcBF-78KbLXRpHGJ4mnIhtfA4Jx8ke47YIE62Quh6yW_Uwznw=s256"
},
{
"title": "Stranger Than Fiction",
"link": "https://play.google.com/store/movies/details/Stranger_Than_Fiction?id=C4pDYO8HlaA.P",
"product_id": "C4pDYO8HlaA.P",
"video": "https://play.google.com/video/lava/web/player/yt:movie:CwRjNOF-ex8.P?autoplay=1&embed=play",
"price": "$3.99",
"extracted_price": 3.99,
"thumbnail": "https://play-lh.googleusercontent.com/cDe2u0I_A0LG8Rr1ogBPzVfdqOzD3OiYATRuLBnR8vEiA0moRXHEcRU0krddPZTb2egt9nbW-ElFpsRUgDY=s256"
}
]
}
]
}
Books Store - Search query for only paid books
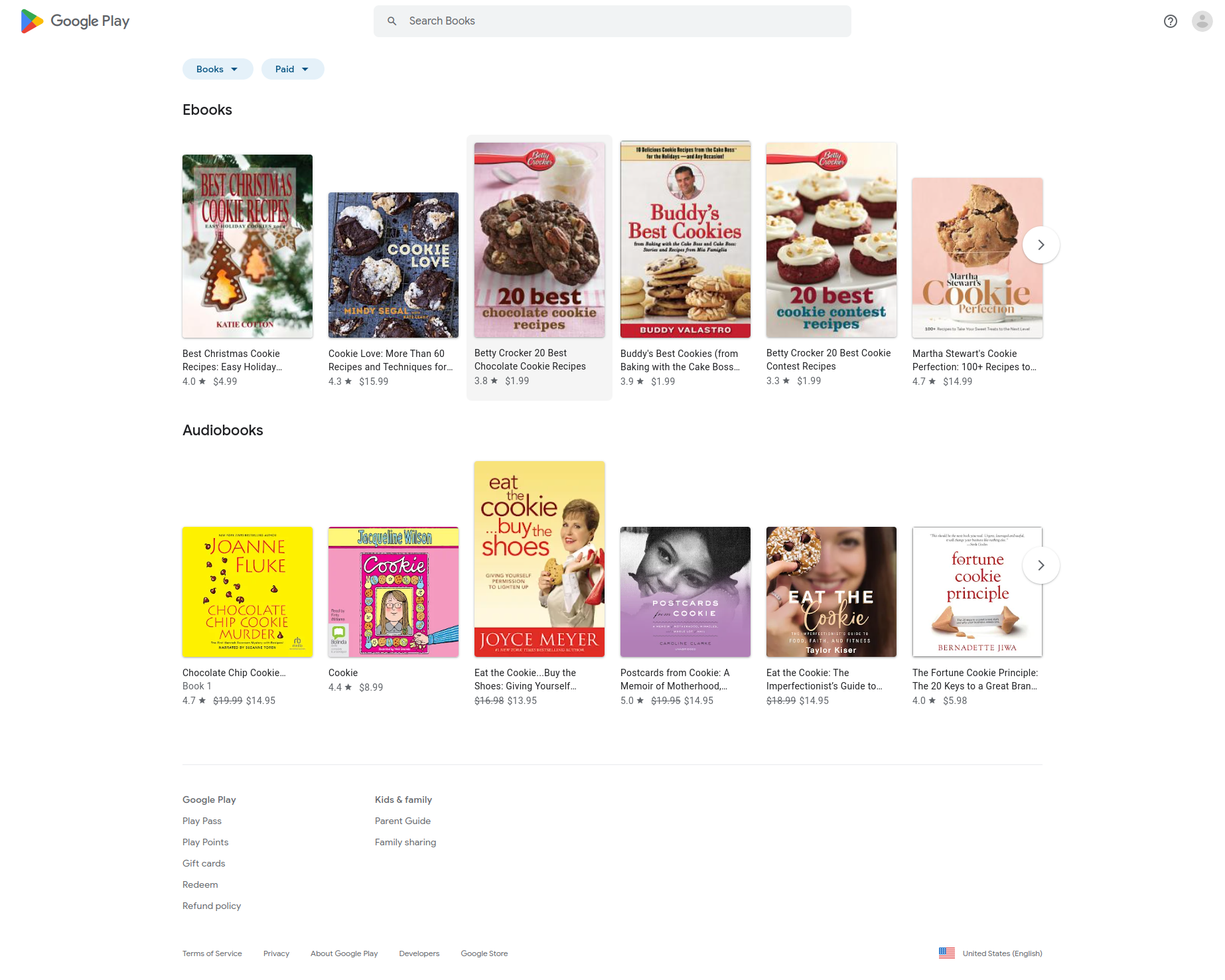
https://www.searchapi.io/api/v1/search?engine=google_play_store&price=2&q=Cookie&store=books
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_play_store",
"store": "books",
"q": "Cookie",
"price": "2"
}
response = requests.get(url, params=params)
print(response.text)
{
"organic_results": [
{
"title": "Ebooks",
"items": [
{
"title": "Best Christmas Cookie Recipes: Easy Holiday Cookies 2014",
"link": "https://play.google.com/store/books/details/Katie_Cotton_Best_Christmas_Cookie_Recipes?id=hbroBAAAQBAJ",
"product_id": "hbroBAAAQBAJ",
"rating": 4.0,
"price": "$4.99",
"extracted_price": 4.99,
"thumbnail": "https://books.google.com/books/publisher/content/images/frontcover/hbroBAAAQBAJ?fife=w256-h256"
},
{
"title": "Cookie Love: More Than 60 Recipes and Techniques for Turning the Ordinary into the Extraordinary [A Baking Book]",
"link": "https://play.google.com/store/books/details/Mindy_Segal_Cookie_Love?id=KtqWBAAAQBAJ",
"product_id": "KtqWBAAAQBAJ",
"rating": 4.3,
"price": "$15.99",
"extracted_price": 15.99,
"thumbnail": "https://books.google.com/books/publisher/content/images/frontcover/KtqWBAAAQBAJ?fife=w256-h256"
},
{
"title": "Betty Crocker 20 Best Chocolate Cookie Recipes",
"link": "https://play.google.com/store/books/details/Betty_Crocker_Betty_Crocker_20_Best_Chocolate_Cook?id=BJRbvt2-R-wC",
"product_id": "BJRbvt2-R-wC",
"rating": 3.8,
"price": "$1.99",
"extracted_price": 1.99,
"thumbnail": "https://books.google.com/books/content/images/frontcover/BJRbvt2-R-wC?fife=w256-h256"
}
]
}
],
"pagination": {
"next_page_token": "Cgj6noGdAwIIFBAUGhOiARAKBkNvb2tpZRgBKgIIAjBAOhAKCAoGQ29va2llEAAYAiAB"
}
}